JavaScript: Nth Tetrahedral Number
JavaScript Math: Exercise-78 with Solution
Nth Tetrahedral Number
Write a JavaScript program that takes an integer n and returns the nth Tetrahedral number.
A tetrahedral number, or triangular pyramidal number, is a figurate number that represents a pyramid with a triangular base and three sides, called a tetrahedron. The formula for the nth tetrahedral number is represented by the 3rd rising factorial of n divided by the factorial of 3:
The tetrahedral numbers are:
1, 4, 10, 20, 35, 56, 84, 120, 165, 220, ...
Test Data:
(1) -> 1
(2) -> 4
(3) -> 10
(4) -> 20
(5) -> 35
Sample Solution:
JavaScript Code:
/**
* Function to calculate the nth Tetrahedral number.
* @param {number} n - The input number.
* @returns {number} - The nth Tetrahedral number.
*/
function test(n) {
// Calculate the Tetrahedral number using the formula (n * (n + 1) * (n + 2)) / 6
return n * (n + 1) * (n + 2) / 6;
}
// Test cases
// Assign value to n
n = 1;
// Print n
console.log("n = " + n);
// Print nth Tetrahedral number
console.log("nth Tetrahedral number = " + test(n));
// Repeat the above steps for different values of n
n = 2;
console.log("n = " + n);
console.log("nth Tetrahedral number = " + test(n));
n = 3;
console.log("n = " + n);
console.log("nth Tetrahedral number = " + test(n));
n = 4;
console.log("n = " + n);
console.log("nth Tetrahedral number = " + test(n));
n = 5;
console.log("n = " + n);
console.log("nth Tetrahedral number = " + test(n));
Output:
n = 1 nth Tetrahedral number = 1 n = 2 nth Tetrahedral number = 4 n = 3 nth Tetrahedral number = 10 n = 4 nth Tetrahedral number = 20 n = 5 nth Tetrahedral number = 35
Flowchart:
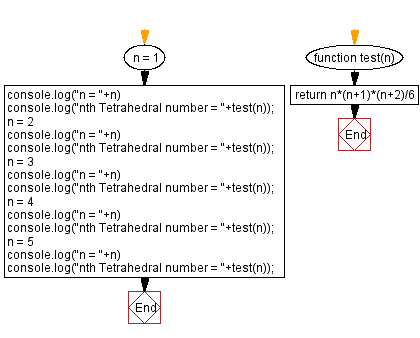
Live Demo:
See the Pen javascript-math-exercise-78 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that calculates the nth tetrahedral number using the formula n(n+1)(n+2)/6.
- Write a JavaScript function that computes tetrahedral numbers iteratively and validates that n is a positive integer.
- Write a JavaScript function that uses recursion to compute the nth tetrahedral number with proper base cases.
- Write a JavaScript function that formats the result of the tetrahedral number calculation to a fixed number of decimals.
Improve this sample solution and post your code through Disqus.
Previous: Two positive integers as string, return their sum.
Next: Sequence number from the given Triangular Number.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.