JavaScript: Subtract without arithmetic operators
JavaScript Math: Exercise-76 with Solution
Write a JavaScript program to subtract one integer from another, without using arithmetic operators such as -, %, /, +, etc.
Test Data:
(200, 100) -> 100
(200, 300) -> -100
(-200, -300) -> 100
(200, 200) -> 0
Sample Solution:
JavaScript Code:
/**
* Performs addition operation using bitwise operators without using arithmetic operators.
* @param {number} a - The first number.
* @param {number} b - The second number.
* @returns {number} - The result of the addition operation.
*/
function test1(a, b)
{
// Execute the loop until b becomes 0
do
{
// Calculate the carry and store it in temp
let temp = (~a) & b;
// Perform bitwise XOR operation to find the sum of a and b and store it in a
a = a ^ b;
// Left shift the carry by 1 and store it in b
b = temp << 1;
}
while (b != 0) // Continue the loop until b becomes 0
// Return the result after addition
return a;
}
// Test cases
console.log(test1(200, 100));
console.log(test1(200, 300));
console.log(test1(-200, -300));
console.log(test1(200, 200));
Output:
100 -100 100 0
Flowchart:
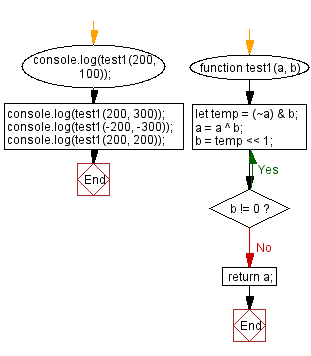
Live Demo:
See the Pen javascript-math-exercise-76 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus.
Previous: Swap three numbers.
Next: Two positive integers as string, return their sum.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/javascript-exercises/javascript-math-exercise-76.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics