JavaScript: Swap three numbers
JavaScript Math: Exercise-75 with Solution
Swap Three Numbers Without Third Variable
Write a JavaScript program to swap three numbers without a third variable.
Test Data:
(100, 200, 300) -> 300, 100, 200
Sample Solution:
JavaScript Code:
/**
* Swaps the values of three variables using arithmetic operations.
* @param {number} x - The first number.
* @param {number} y - The second number.
* @param {number} z - The third number.
* @returns {Array} - An array containing the swapped values of x, y, and z.
*/
function test(x, y, z)
{
// Calculate the sum of x, y, and z and assign it to x
x = x + y + z;
// Calculate y's new value using arithmetic operations
y = x - (y + z);
// Calculate z's new value using arithmetic operations
z = x - (y + z);
// Calculate x's new value using arithmetic operations
x = x - (y + z);
// Return an array containing the swapped values
return [x, y, z];
}
// Initialize variables x, y, and z
x = 100;
y = 200;
z = 300;
// Display the values before swapping
console.log("Before swapping:")
console.log("x = "+x);
console.log("y = "+y);
console.log("z = "+z);
// Call the test function to swap the values and store the result
result = test(x, y, z);
// Display the values after swapping
console.log("After swapping:");
console.log("x = "+result[0]);
console.log("y = "+result[1]);
console.log("z = "+result[2]);
Output:
Before swapping: x = 100 y = 200 z = 300 After swapping: x = 300 y = 100 z = 200
Flowchart:
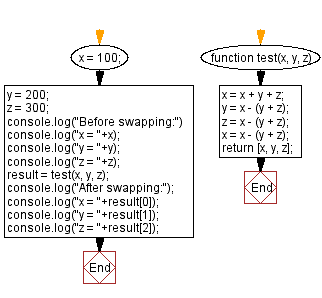
Live Demo:
See the Pen javascript-math-exercise-75 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus.
Previous: Hexadecimal number to binary equivalent.
Next: Subtract without arithmetic operators.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics