JavaScript: Hexadecimal number to binary equivalent
JavaScript Math: Exercise-74 with Solution
Write a JavaScript program to convert a hexadecimal number to its binary equivalent (as a string).
From Wikipedia –
In mathematics and computing, the hexadecimal (also base-16 or simply hex) numeral system is a positional numeral system that represents numbers using a radix (base) of 16. Unlike the decimal system representing numbers using 10 symbols, hexadecimal uses 16 distinct symbols, most often the symbols "0"–"9" to represent values 0 to 9, and "A"–"F" (or alternatively "a"–"f") to represent values from 10 to 15.
A binary number is a number expressed in the base-2 numeral system or binary numeral system, a method of mathematical expression which uses only two symbols: typically "0" (zero) and "1" (one).
Test Data:
("7B316") -> "1111011001100010110"
("6F16") -> "110111100010110"
("4B2A16") -> "10010110010101000010110"
Sample Solution:
JavaScript Code:
/**
* Converts a hexadecimal number to its binary representation.
* @param {string} n - The hexadecimal number to convert.
* @returns {string} - The binary representation of the hexadecimal number.
*/
function test(n){
// Parse the hexadecimal number to an integer, then convert it to binary and pad it with leading zeros to ensure it has 8 digits
return (parseInt(n, 16).toString(2)).padStart(8, '0');
}
// Test the function with different hexadecimal numbers
console.log(test("7B316"));
console.log(test("6F16"));
console.log(test("4B2A16"));
Output:
1111011001100010110 110111100010110 10010110010101000010110
Flowchart:
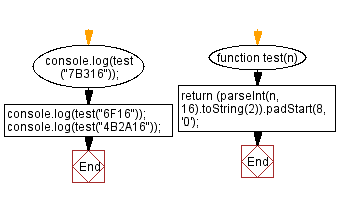
Live Demo:
See the Pen javascript-math-exercise-74 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus.
Previous: Check sum of consecutive positive integers.
Next: Swap three numbers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/javascript-exercises/javascript-math-exercise-74.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics