JavaScript: Check sum of consecutive positive integers
JavaScript Math: Exercise-73 with Solution
Write a JavaScript program to check if a given positive integer can be expressed as the sum of two or more consecutive positive integers.
Sample Data:
33 can be represented as 11 + 22
10 = 1+2+3+4
but 8 cannot be represented in this way.
Sample Solution-1:
JavaScript Code:
/**
* Checks if a given number is not a power of two.
* @param {number} n - The number to check.
* @returns {boolean} - True if the number is not a power of two, false otherwise.
*/
function test(n)
{
// Perform bitwise AND operation between n and n - 1, and check if the result is not zero
return (n & (n - 1)) != 0;
}
// Test the function with different numbers
console.log(test(33)); // Output: true
console.log(test(10)); // Output: true
console.log(test(8)); // Output: false
Output:
true true false
Flowchart:
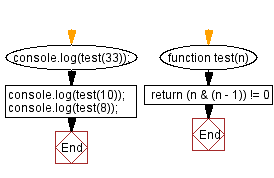
Live Demo:
See the Pen javascript-math-exercise-73-1 by w3resource (@w3resource) on CodePen.
Sample Solution-2:
JavaScript Code:
/**
* Checks if a given number is not a power of two.
* @param {number} n - The number to check.
* @returns {boolean} - True if the number is not a power of two, false otherwise.
*/
function test(n){
// Calculate the base-2 logarithm of n and check if it's not an integer
return !Number.isInteger(Math.log2(n));
}
// Test the function with different numbers
console.log(test(33)); // Output: true
console.log(test(10)); // Output: true
console.log(test(8)); // Output: false
Output:
true true false
Flowchart:
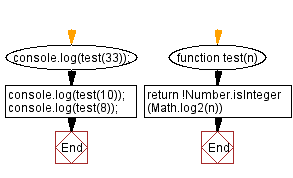
Live Demo:
See the Pen javascript-math-exercise-73-2 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus.
Previous: JavaScript Pronic Number.
Next: Hexadecimal number to binary equivalent
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/javascript-exercises/javascript-math-exercise-73.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics