JavaScript: All prime factors of a given number
JavaScript Math: Exercise-71 with Solution
Prime Factors of a Number
Write a JavaScript program to print all the prime factors of a given number.
Visualisation:
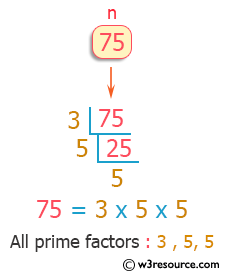
Test Data:
(75) -> [3, 5, 5]
(18) -> [2, 3, 3]
(101) -> [101]
Sample Solution:
JavaScript Code:
Output:
[3,5,5] [2,3,3] [101]
Flowchart:
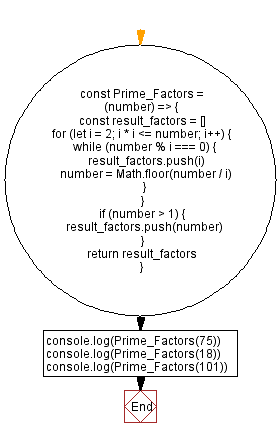
Live Demo:
For more Practice: Solve these Related Problems:
- Write a JavaScript function that returns an array of prime factors for a given integer using iterative trial division.
- Write a JavaScript function that recursively factors a number into its prime constituents and returns them in sorted order.
- Write a JavaScript function that validates input and returns an error if the number is not a positive integer before factoring.
- Write a JavaScript function that factors a number and also counts the multiplicity of each prime factor in the result.
Improve this sample solution and post your code through Disqus.
Previous: Reverse Polish notation in mathematical expression.
Next: JavaScript Pronic number.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.