JavaScript: Find the lowest value in an array
JavaScript Math: Exercise-7 with Solution
Find Minimum in Array
Write a JavaScript function to find the lowest value in an array.
Test Data :
console.log(min([12,34,56,1]));
console.log(min([-12,-34,0,-56,-1]));
1
-56
Visual Presentation:
Sample Solution:
JavaScript Code:
// Define a function named min that takes an input array.
function min(input) {
// Check if the input is an array, if not, return false.
if (toString.call(input) !== "[object Array]")
return false;
// Return the minimum value from the input array using Math.min.apply.
return Math.min.apply(null, input);
}
// Output the minimum value from the array [12, 34, 56, 1] to the console.
console.log(min([12, 34, 56, 1]));
// Output the minimum value from the array [-12, -34, 0, -56, -1] to the console.
console.log(min([-12, -34, 0, -56, -1]));
Output:
1 -56
Flowchart:
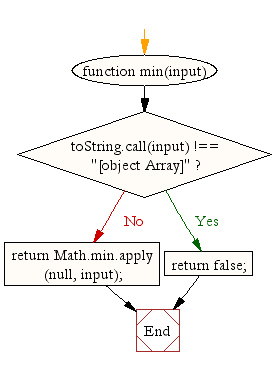
Live Demo:
See the Pen javascript-math-exercise-7 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that finds the minimum number in an array using recursion without built-in functions.
- Write a JavaScript function that iterates over an array to determine the smallest number using conditional checks.
- Write a JavaScript function that filters out non-numeric values before computing the minimum in an array.
- Write a JavaScript function that applies the reduce() method to identify the smallest number in an array.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript function to find the highest value in an array.
Next: Write a JavaScript function to get the greatest common divisor (gcd) of two integers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.