JavaScript: Twin prime of a prime number
JavaScript Math: Exercise-66 with Solution
Find Twin Prime
Write a JavaScript program to find the 'twin prime' of a given prime number.
From Wikipedia,
A twin prime is a prime number that is either 2 less or 2 more than another prime number-for example, either member of the twin prime pair (41, 43). In other words, a twin prime is a prime that has a prime gap of two. Sometimes the term twin prime is used for a pair of twin primes; an alternative name for this is prime twin or prime pair.
Usually the pair (2, 3) is not considered to be a pair of twin primes. Since 2 is the only even prime, this pair is the only pair of prime numbers that differ by one; thus twin primes are as closely spaced as possible for any other two primes.
The first few twin prime pairs are:
(3, 5), (5, 7), (11, 13), (17, 19), (29, 31), (41, 43), (59, 61), (71, 73), (101, 103), (107, 109), (137, 139), ....
Return value: Either the twin, or -1 if n or n + 2 is not prime number.
Sample Data:
Twin prime of 5 is:7
Twin prime of 59 is:61
Twin prime of 107 is:109
Twin prime of 61 is:-1
Sample Solution:
JavaScript Code:
// Define a function twin_Prime to find the twin prime for a given number.
function twin_Prime (n) {
// Call the test_prime function to check if 'n' is prime.
const prime_num = test_prime(n);
// If 'n' is not prime, return -1.
if (!prime_num) {
return -1;
}
// If 'n' is prime, check if 'n+2' is also prime.
if (!test_prime(n + 2)) {
return -1;
}
// Return 'n+2' if it is prime and forms a twin prime pair with 'n'.
return n + 2;
}
// Define a function test_prime to check if a number is prime.
function test_prime(n)
{
// If 'n' is 1, it is not prime.
if (n === 1)
{
return false;
}
// If 'n' is 2, it is prime.
else if(n === 2)
{
return true;
}
// For 'n' greater than 2, iterate from 2 to 'n-1' to check for factors.
else
{
for(var x = 2; x < n; x++)
{
// If 'n' is divisible by any number between 2 and 'n-1', it is not prime.
if(n % x === 0)
{
return false;
}
}
// If 'n' is not divisible by any number between 2 and 'n-1', it is prime.
return true;
}
}
// Output the twin primes for specific numbers.
console.log("Twin prime of 5 is:" + twin_Prime(5));
console.log("Twin prime of 59 is:" + twin_Prime(59));
console.log("Twin prime of 107 is:" + twin_Prime(107));
console.log("Twin prime of 61 is:" + twin_Prime(61));
Output:
"Twin prime of 5 is:7" "Twin prime of 59 is:61" "Twin prime of 107 is:109" "Twin prime of 61 is:-1"
Flowchart:
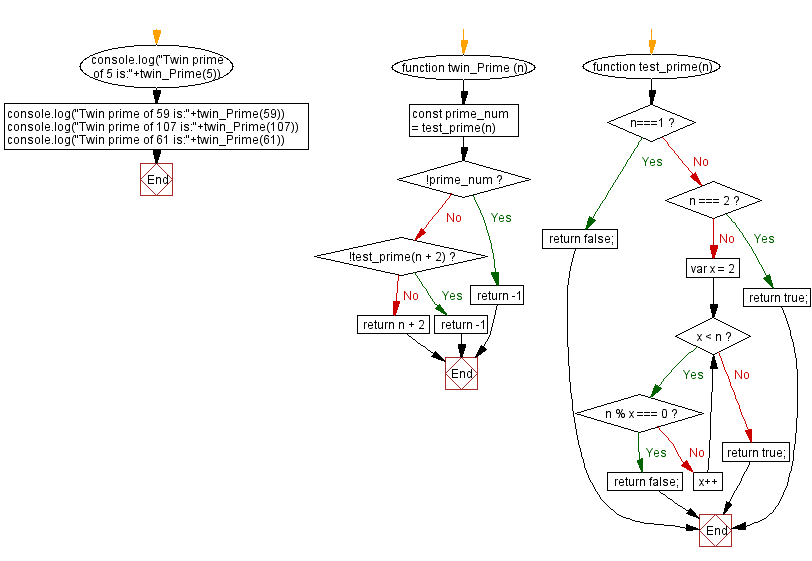
Live Demo:
See the Pen javascript-math-exercise-66 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that finds the twin prime of a given prime number by checking numbers two less and two more.
- Write a JavaScript function that validates a prime number input and returns its twin prime pair if one exists.
- Write a JavaScript function that iterates through a range of primes to identify and return the first twin prime pair after a given number.
- Write a JavaScript function that uses efficient prime checking algorithms to find twin primes in a specified range.
Improve this sample solution and post your code through Disqus.
Previous: Find Lucas number from index value.
Next: Sum of a geometric progression.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.