JavaScript: Find Lucas number from index value
JavaScript Math: Exercise-65 with Solution
Nth Lucas Number
Write a JavaScript program to get the Nth Lucas Number.
From Wikipedia-
The Lucas numbers or Lucas series are an integer sequence named after the mathematician Francois Edouard Anatole Lucas (1842-1891), who studied both that sequence and the closely related Fibonacci numbers. Lucas numbers and Fibonacci numbers form complementary instances of Lucas sequences.
The Lucas series has the same recursive relationship as the Fibonacci sequence, where each term is the sum of the two previous terms, but with different starting values. This produces a sequence where the ratios of successive terms approach the golden ratio, and in fact the terms themselves are roundings of integer powers of the golden ratio. The sequence also has a variety of relationships with the Fibonacci numbers, like the fact that adding any two Fibonacci numbers two terms apart in the Fibonacci sequence results in the Lucas number in between.
The first few Lucas numbers are:
2, 1, 3, 4, 7, 11, 18, 29, 47, 76, 123, 199, 322, 521, 843, 1364, 2207, 3571, 5778, 9349 ....
.
Sample Data:
0 -> 2
4 -> 7
7 -> 29
Sample Solution:
JavaScript Code:
// Define a function find_lucas_number that calculates the Lucas number at a given index.
function find_lucas_number(index) {
// Check if the index is non-negative.
if (index >= 0) {
// Check if the index is an integer.
if (Math.floor(index) !== index) throw new TypeError('Index cannot be a Decimal')
let x = 2;
let y = 1;
// Iterate through the Lucas series to find the Lucas number at the given index.
for (let i = 0; i < index; i++) {
const temp = x + y;
x = y;
y = temp;
}
return x;
}
}
// Call the find_lucas_number function with valid and invalid arguments and output the results.
console.log(find_lucas_number(0));
console.log(find_lucas_number(4));
console.log(find_lucas_number(7));
console.log(find_lucas_number(5.4));
Output:
2 7 29 -------------------------------------------------- Uncaught TypeError: Index cannot be a Decimal at https://cdpn.io/cpe/boomboom/pen.js?key=pen.js-fb7a2744-ca9f-8c12-e963-385a69e41cff:6
Flowchart:
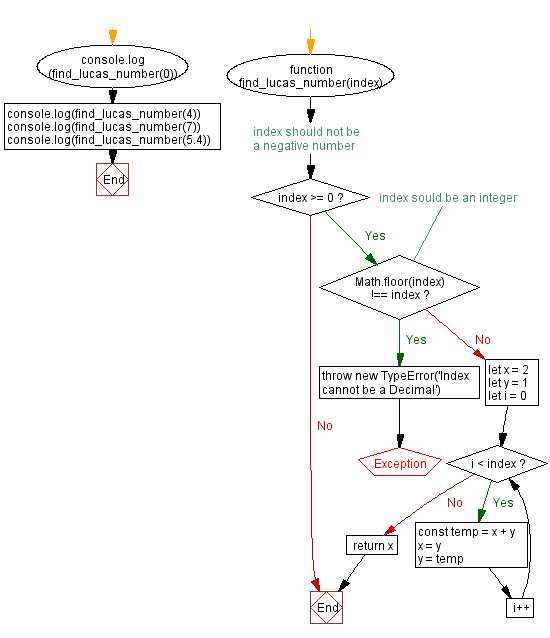
Live Demo:
See the Pen javascript-math-exercise-65 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that computes the nth Lucas number recursively with memoization for optimization.
- Write a JavaScript function that calculates the nth Lucas number iteratively and handles large inputs efficiently.
- Write a JavaScript function that compares Lucas numbers with Fibonacci numbers for the same index and returns both.
- Write a JavaScript function that computes the nth Lucas number and returns the result in scientific notation if necessary.
Improve this sample solution and post your code through Disqus.
Previous: Volume of a Hemisphere.
Next: Twin prime of a prime number
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.