JavaScript: Volume of a Pentagonal Prism
JavaScript Math: Exercise-62 with Solution
Volume of a Prism with Hexagonal Side
Write a JavaScript program to calculate the volume of a prism using only its height and one of its hexagonal sides.
In geometry, the pentagonal prism is a prism with a pentagonal base. It is a type of heptahedron with seven faces, fifteen edges, and ten vertices.
Sample Data:
Sample Solution:
JavaScript Code:
// Define a function volume_Pentagonal_Prism that calculates the volume of a pentagonal prism given its base edge and height.
const volume_Pentagonal_Prism = (base_edge, height) => {
// Check if the base edge and height are numbers and positive.
is_Number(base_edge, 'Base Edge');
is_Number(height, 'Height');
// Return the volume of the pentagonal prism.
return (1/4 * height * base_edge * base_edge * Math.sqrt(5 * (5 + 2 * (Math.sqrt(5)))));
}
// Define a function is_Number that checks if a given value is a number and positive.
const is_Number = (n, n_name = 'number') => {
// If the given value is not a number, throw a TypeError.
if (typeof n !== 'number') {
throw new TypeError('The ' + n_name + ' is not Number type!');
}
// If the given value is negative or not a finite number, throw an Error.
else if (n < 0 || (!Number.isFinite(n))) {
throw new Error('The ' + n_name + ' must be a positive values!');
}
}
// Call the volume_Pentagonal_Prism function with valid and invalid arguments and output the results.
console.log(volume_Pentagonal_Prism(4.0, 8.0));
console.log(volume_Pentagonal_Prism('4.0', 8.0));
console.log(volume_Pentagonal_Prism(4.0, -8.0));
Output:
220.22110727538777 ----------------------------------------------------------- Uncaught TypeError: The Base Edge is not Number type! at https://cdpn.io/cpe/boomboom/pen.js?key=pen.js-5d61fb21-ef72-d840-7127-0d851f83a866:9 ----------------------------------------------------------------------------------------- Uncaught Error: The Height must be a positive values! at https://cdpn.io/cpe/boomboom/pen.js?key=pen.js-efb93b94-2200-1f40-7134-9a617d9067bc:13
Flowchart:
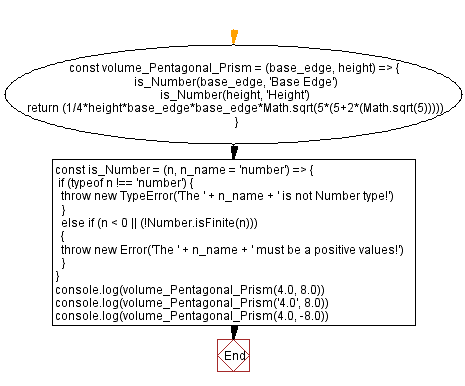
Live Demo:
See the Pen javascript-math-exercise-62 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that calculates the volume of a prism with a hexagonal base given one side length and the height.
- Write a JavaScript function that computes the volume of a hexagonal prism and validates the input for positivity.
- Write a JavaScript function that calculates the volume of a hexagonal prism and returns the result rounded to two decimals.
- Write a JavaScript function that compares the volume of a hexagonal prism to that of a rectangular prism with similar dimensions.
Improve this sample solution and post your code through Disqus.
Previous: Volume of a Triangular Prism.
Next: Volume of a Sphere
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.