JavaScript: Volume of a Pyramid
JavaScript Math: Exercise-59 with Solution
Volume of a Pyramid
Write a JavaScript program to calculate the volume of a Pyramid.
In geometry, a pyramid is a polyhedron formed by connecting a polygonal base and a point, called the apex. Each base edge and apex form a triangle, called a lateral face. It is a conic solid with polygonal base. A pyramid with an n-sided base has n + 1 vertices, n + 1 faces, and 2n edges. All pyramids are self-dual.
Sample Solution:
JavaScript Code:
// Define a function volume_Pyramid that calculates the volume of a pyramid given its base length, base width, and height.
const volume_Pyramid = (baseLength, baseWidth, height) => {
// Check if baseLength, baseWidth, and height are numbers and positive.
is_Number(baseLength, 'BaseLength');
is_Number(baseWidth, 'BaseWidth');
is_Number(height, 'Height');
// Return the volume of the pyramid.
return (baseLength * baseWidth * height) / 3.0;
}
// Define a function is_Number that checks if a given value is a number and positive.
const is_Number = (n, n_name = 'number') => {
// If the given value is not a number, throw a TypeError.
if (typeof n !== 'number') {
throw new TypeError('The ' + n_name + ' is not Number type!');
}
// If the given value is negative or not a finite number, throw an Error.
else if (n < 0 || (!Number.isFinite(n))) {
throw new Error('The ' + n_name + ' must be a positive values!');
}
}
// Call the volume_Pyramid function with valid and invalid arguments and output the results.
console.log(volume_Pyramid(2.0, 3.0, 7.0));
console.log(volume_Pyramid(2.0, 3.0, '7.0'));
console.log(volume_Pyramid(2.0, -3.0, 7.0));
Output:
14 -------------------------------------------------- Uncaught TypeError: The Height is not Number type! at https://cdpn.io/cpe/boomboom/pen.js?key=pen.js-968300e8-d91d-a969-99b2-b16641f784d8:9 ------------------------------------------------------------------------------------------- Uncaught Error: The BaseWidth must be a positive values! at https://cdpn.io/cpe/boomboom/pen.js?key=pen.js-6c222a2a-2e46-07e7-74dd-cd13634303e6:11
Flowchart:
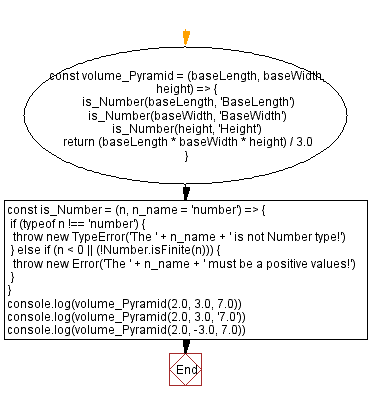
Live Demo:
See the Pen javascript-math-exercise-59 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that calculates the volume of a pyramid using the formula (1/3)*base_area*height and checks for valid inputs.
- Write a JavaScript function that computes the volume of a pyramid and returns additional details like the number of faces.
- Write a JavaScript function that calculates pyramid volume and supports different base shapes by taking the area as an argument.
- Write a JavaScript function that formats the calculated pyramid volume to a fixed number of decimals for presentation.
Improve this sample solution and post your code through Disqus.
Previous: Volume of a Cone.
Next: Volume of a Cylinder
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.