JavaScript: Multiply two complex numbers
JavaScript Math: Exercise-52 with Solution
Multiply Complex Numbers
Write a JavaScript program to multiply two complex numbers.
A complex number is a number that can be expressed in the form a + bi, where a and b are real numbers and i is the imaginary unit, that satisfies the equation i2 = −1. In this expression, a is the real part and b is the imaginary part of the complex number.
Sample Solution:
JavaScript Code:
// Define a constructor function named Complex that represents complex numbers with real and imaginary parts.
function Complex(real, imaginary) {
// Initialize real and imaginary parts of the complex number.
this.real = 0;
this.imaginary = 0;
// Set the real part to the provided value or default to 0 if not provided.
this.real = (typeof real === 'undefined') ? this.real : parseFloat(real);
// Set the imaginary part to the provided value or default to 0 if not provided.
this.imaginary = (typeof imaginary === 'undefined') ? this.imaginary : parseFloat(imaginary);
}
// Define a static method named transform for the Complex function to transform numbers to complex numbers.
Complex.transform = function(num) {
var complex;
// Check if the provided number is already a Complex instance.
complex = (num instanceof Complex) ? num : complex;
// Check if the provided number is a real number and convert it to a complex number with imaginary part 0.
complex = (typeof num === 'number') ? new Complex(num, 0) : num;
// Return the complex number.
return complex;
};
// Define a function named display_complex to display complex numbers in a readable format.
function display_complex(re, im) {
// If the imaginary part is 0, return only the real part.
if(im === '0') return '' + re;
// If the real part is 0, return only the imaginary part with 'i'.
if(re === 0) return '' + im + 'i';
// If the imaginary part is negative, return the real and imaginary parts combined with 'i'.
if(im < 0) return '' + re + im + 'i';
// Otherwise, return the real and imaginary parts combined with '+' and 'i'.
return '' + re + '+' + im + 'i';
}
// Define a function named complex_num_multiply to multiply two complex numbers.
function complex_num_multiply(first, second) {
// Transform the input numbers to complex numbers.
var num1, num2;
num1 = Complex.transform(first);
num2 = Complex.transform(second);
// Calculate the real and imaginary parts of the product.
var real = (num1.real * num2.real) - (num1.imaginary * num2.imaginary);
var imaginary = (num1.real * num2.imaginary) + (num1.imaginary * num2.real);
// Display the result as a complex number.
return display_complex(real, imaginary);
}
// Create two complex numbers.
var a = new Complex(2, -7);
var b = new Complex(4, 3);
// Output the result of multiplying the two complex numbers.
console.log(complex_num_multiply(a,b));
Output:
29-22i
Flowchart:
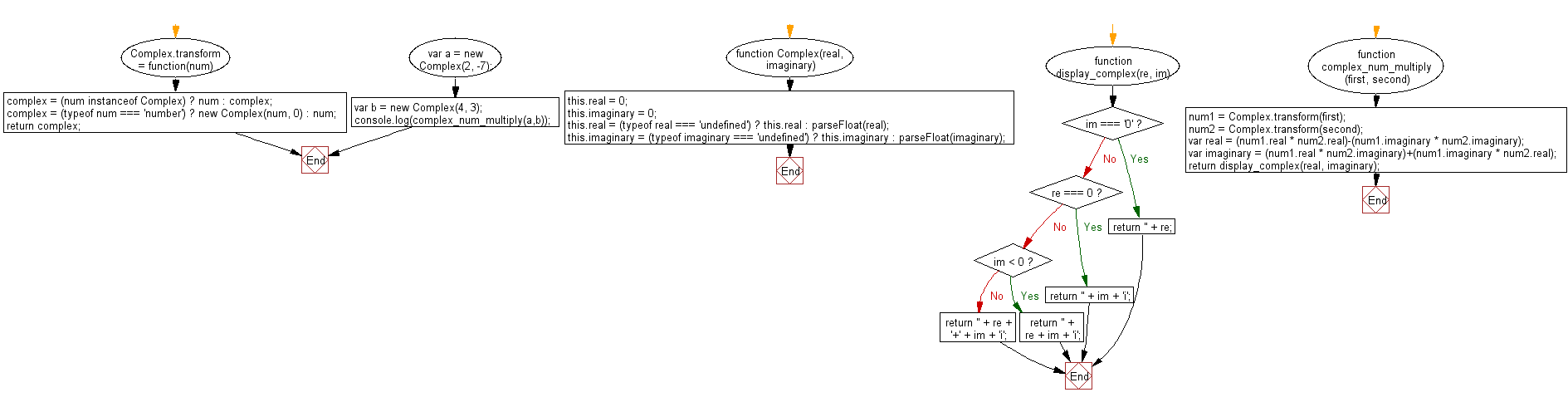
Live Demo:
See the Pen javascript-math-exercise-52 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that multiplies two complex numbers using the formula (a+bi)*(c+di) and returns the product as an object.
- Write a JavaScript function that multiplies complex numbers and outputs the result in both rectangular and polar forms.
- Write a JavaScript function that validates complex number objects before performing multiplication and handles special cases.
- Write a JavaScript function that implements complex multiplication using string parsing and returns a formatted result.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript program to subtract two complex numbers.
Next: Write a JavaScript program to divide two complex numbers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.