JavaScript: Format a number up to specified decimal places
JavaScript Math: Exercise-5 with Solution
Format Number to Decimal Places
Write a JavaScript function to format a number up to specified decimal places.
Test Data :
console.log(decimals(2.100212, 2));
console.log(decimals(2.100212, 3));
console.log(decimals(2100, 2));
"2.10"
"2.100"
"2100.00"
Visual Presentation:
Sample Solution:
JavaScript Code:
// Define a function named decimals that takes two parameters: n (number) and d (number of decimal places).
function decimals(n, d) {
// Check if both n and d are of type number, otherwise return false.
if ((typeof n !== 'number') || (typeof d !== 'number'))
return false;
// Convert n to a floating-point number, defaulting to 0 if conversion fails.
n = parseFloat(n) || 0;
// Return the number n formatted to have d decimal places using the toFixed method.
return n.toFixed(d);
}
// Output the result of formatting the number 2.100212 with 2 decimal places to the console.
console.log(decimals(2.100212, 2));
// Output the result of formatting the number 2.100212 with 3 decimal places to the console.
console.log(decimals(2.100212, 3));
// Output the result of formatting the number 2100 with 2 decimal places to the console.
console.log(decimals(2100, 2));
Output:
2.10 2.100 2100.00
Explanation:
In the exercise above,
- The code defines a function called "decimals()" which takes two parameters: 'n' (a number) and 'd' (the number of decimal places to format).
- Inside the function:
- It first checks whether both 'n' and 'd' are of type number. If either of them is not a number, it returns 'false'.
- It then converts the input 'n' into a floating-point number using 'parseFloat', defaulting to 0 if the conversion fails.
- The function then uses the "toFixed()" method to format the number n to have 'd' decimal places.
Flowchart:
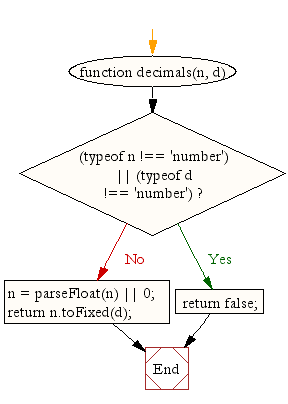
Live Demo:
See the Pen javascript-math-exercise-5 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that formats a number to a specified number of decimal places without using toFixed().
- Write a JavaScript function that rounds a number to a given number of decimal places and returns a string with trailing zeros if needed.
- Write a JavaScript function that handles formatting edge cases, such as very small numbers or numbers expressed in scientific notation.
- Write a JavaScript function that formats both positive and negative numbers to a specified number of decimal places consistently.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript function to generate a random integer.
Next: Write a JavaScript function to find the highest value in an array.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics