JavaScript: Subtract elements from one another in an array
JavaScript Math: Exercise-45 with Solution
Subtract Elements from Array
Write a JavaScript function to subtract elements from an array.
Sample Solution:
JavaScript Code:
// Define a function named subtraction that performs subtraction on an array of numbers.
function subtraction(arr)
{
// Check if the input is an array.
if (Object.prototype.toString.call(arr) === '[object Array]') {
// Initialize the total variable with the first element of the array.
var total = arr[0];
// Check if the first element is a number.
if (typeof (total) !== 'number') {
return false;
}
// Iterate through the array starting from the second element.
for (var i = 1, length = arr.length; i < length; i++)
{
// Check if the current element is a number.
if (typeof (arr[i]) === 'number')
{
// Subtract the current element from the total.
total -= arr[i];
}
else
return false;
}
// Return the result of the subtraction.
return total;
}
else
return false;
}
// Output the result of subtracting elements in the array [7, 3, 2, -1] to the console.
console.log(subtraction([7,3, 2,-1]));
Output:
3
Visual Presentation:
Flowchart:
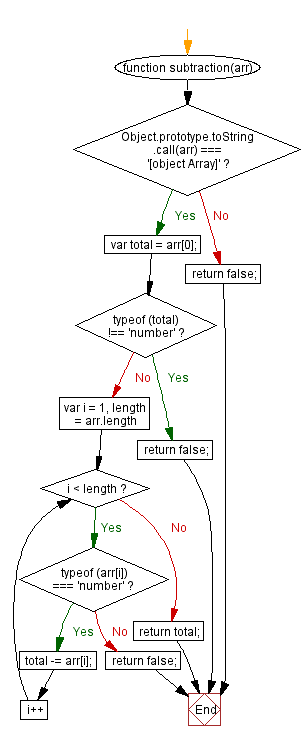
Live Demo:
See the Pen javascript-math-exercise-45 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript function to show the first twenty Hamming numbers.
Next: Write a JavaScript function to calculate the divisor and modulus of two integers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics