JavaScript: Show the Hamming numbers
JavaScript Math: Exercise-44 with Solution
Generate First 20 Hamming Numbers
Write a JavaScript function to show the first twenty Hamming numbers. Hamming numbers are numbers with prime factors of 2, 3 and 5
Sample Solution:
JavaScript Code:
// Define a function named Hamming that generates a sequence of Hamming numbers up to the nth number.
function Hamming(n) {
// Initialize an array to store the Hamming numbers.
var succession = [1];
// Initialize a variable to store the length of the succession array.
var length = succession.length;
// Initialize a variable to store the candidate number starting from 2.
var candidate = 2;
// Continue the loop until the length of the succession array reaches 'n'.
while (length < n) {
// If the candidate number is a Hamming number, add it to the succession array.
if (isHammingNumber(candidate)) {
succession[length] = candidate;
length++;
}
// Move to the next candidate number.
candidate++;
}
// Return the array of Hamming numbers.
return succession;
}
// Define a helper function named isHammingNumber that checks if a number is a Hamming number.
function isHammingNumber(num) {
// Divide the number by 5 repeatedly until it is no longer divisible by 5.
while (num % 5 === 0) num /= 5;
// Divide the number by 3 repeatedly until it is no longer divisible by 3.
while (num % 3 === 0) num /= 3;
// Divide the number by 2 repeatedly until it is no longer divisible by 2.
while (num % 2 === 0) num /= 2;
// Check if the remaining number is 1 (a Hamming number).
return num == 1;
}
// Output the result of generating the first 20 Hamming numbers to the console.
console.log(Hamming(20));
Output:
[1,2,3,4,5,6,8,9,10,12,15,16,18,20,24,25,27,30,32,36]
Visual Presentation:
Flowchart:
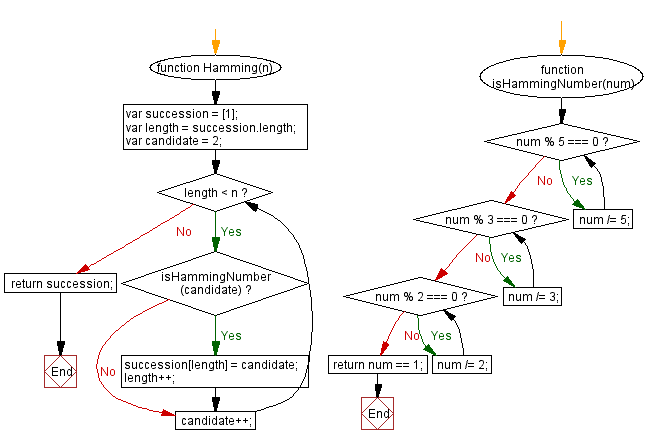
Live Demo:
See the Pen javascript-math-exercise-44 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that generates the first 20 Hamming numbers using a heap-based approach.
- Write a JavaScript function that iteratively computes Hamming numbers and stores them in an array until 20 numbers are obtained.
- Write a JavaScript function that uses multiple pointers to merge multiples of 2, 3, and 5 into a sequence of Hamming numbers.
- Write a JavaScript function that verifies each Hamming number by checking its prime factorization before adding it to the list.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript function to get all prime numbers from 0 to a specified number
Next: Write a JavaScript function to subtract elements from one another in an array.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.