JavaScript: Calculate the combination of n and r
JavaScript Math: Exercise-42 with Solution
Calculate Combination (nCr)
Write a JavaScript function to calculate the combination of n and r.
The formula is : n!/(r!*(n - r)!).
Test Data :
console.log(combinations(6, 2));
15
console.log(combinations(5, 3));
10
Sample Solution:
JavaScript Code:
// Define a function named product_Range that calculates the product of all integers in the range [a, b].
function product_Range(a, b) {
// Initialize the product with the value of 'a'.
var prd = a,
i = a;
// Iterate from 'a' to 'b' (excluding 'b').
while (i++ < b) {
// Multiply the product by the current value of 'i'.
prd *= i;
}
// Return the final product.
return prd;
}
// Define a function named combinations that calculates the number of combinations (n choose r).
function combinations(n, r) {
// Check if either 'n' equals 'r' or 'r' equals 0.
if (n == r || r == 0) {
// If true, return 1.
return 1;
} else {
// If false, determine the larger of (n-r) and 'r' and calculate the combinations using the product_Range function.
r = (r < n - r) ? n - r : r;
return product_Range(r + 1, n) / product_Range(1, n - r);
}
}
// Output the result of calculating combinations(6, 2) to the console.
console.log(combinations(6, 2));
// Output the result of calculating combinations(5, 3) to the console.
console.log(combinations(5, 3));
Output:
15 10
Flowchart:
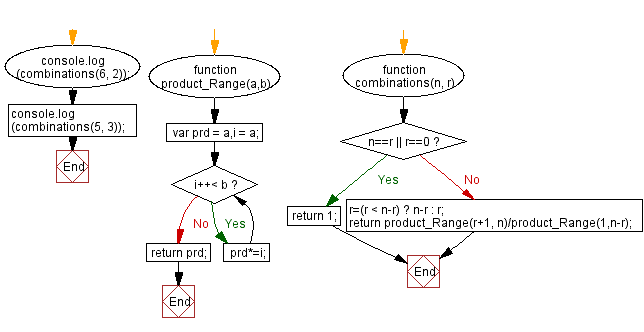
Live Demo:
See the Pen javascript-math-exercise-42 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that calculates nCr recursively using memoization to optimize performance.
- Write a JavaScript function that computes binomial coefficients iteratively by constructing Pascal's triangle.
- Write a JavaScript function that validates inputs for n and r, ensuring they are non-negative integers with r ≤ n.
- Write a JavaScript function that returns the combination as a formatted string, showing the calculation steps.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript function to count the digits of an integer.
Next: Write a JavaScript function to get all prime numbers from 0 to a specified number
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.