JavaScript: Generate a random integer
JavaScript Math: Exercise-4 with Solution
Write a JavaScript function to generate a random integer.
Test Data :
console.log(rand(20,1));
console.log(rand(1,10));
console.log(rand(6));
console.log(rand());
15
5
1
0
Visual Presentation:
Sample Solution:
JavaScript Code:
// Define a function named rand that generates a random integer between the specified minimum and maximum values.
rand = function(min, max) {
// If both minimum and maximum values are not provided, return 0.
if (min == null && max == null)
return 0;
// If only one value is provided, treat it as the maximum and set minimum to 0.
if (max == null) {
max = min;
min = 0;
}
// Generate a random integer between min (inclusive) and max (inclusive).
return min + Math.floor(Math.random() * (max - min + 1));
};
// Output a random integer between 20 and 1 (inclusive) to the console.
console.log(rand(20, 1));
// Output a random integer between 1 and 10 (inclusive) to the console.
console.log(rand(1, 10));
// Output a random integer between 6 and 0 (inclusive) to the console.
console.log(rand(6));
// Output a random integer between 0 and 0 (inclusive) to the console.
console.log(rand());
Output:
4 1 2 0
Explanation:
In the exercise above,
- The code defines a function called "rand()" which generates a random integer within a specified range.
- The "rand()" function takes two parameters: 'min' and 'max', representing the minimum and maximum values of the range.
- Inside the function:
- If both 'min' and 'max' parameters are not provided (null), it returns 0.
- If only one parameter is provided (which is treated as the maximum value), it sets the minimum value to 0.
- It then generates a random integer between 'min' (inclusive) and 'max' (inclusive) using "Math.random()" and some arithmetic operations.
Flowchart:
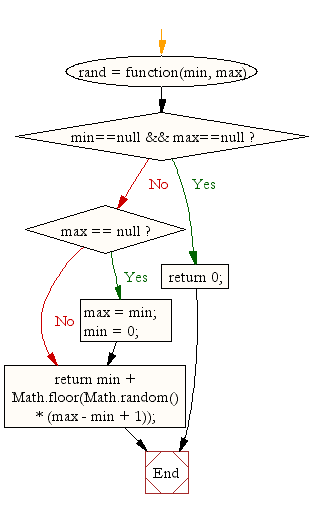
Live Demo:
See the Pen javascript-math-exercise-4 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript function to convert a decimal number to binary, hexadecimal or octal number.
Next: Write a JavaScript function to format a number up to specified decimal places.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/javascript-exercises/javascript-math-exercise-4.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics