JavaScript: Print an integer with commas as thousands separators
JavaScript Math: Exercise-39 with Solution
Format Integer with Thousands Separator
Write a JavaScript function to print an integer with thousands separated by commas.
Test Data:
console.log(thousands_separators(1000));
"1,000"
console.log(thousands_separators(10000.23));
"10,000.23"
console.log(thousands_separators(100000));
"100,000"
Sample Solution:
JavaScript Code:
// Define a function named thousands_separators that adds thousands separators to a number.
function thousands_separators(num)
{
// Convert the number to a string and split it into an array containing the integer part and the decimal part.
var num_parts = num.toString().split(".");
// Add thousands separators to the integer part using a regular expression.
num_parts[0] = num_parts[0].replace(/\B(?=(\d{3})+(?!\d))/g, ",");
// Join the integer and decimal parts back together with a period and return the result.
return num_parts.join(".");
}
// Output the result of adding thousands separators to 1000 to the console.
console.log(thousands_separators(1000));
// Output the result of adding thousands separators to 10000.23 to the console.
console.log(thousands_separators(10000.23));
// Output the result of adding thousands separators to 100000 to the console.
console.log(thousands_separators(100000));
Output:
1,000 10,000.23 100,000
Visual Presentation:
Flowchart:
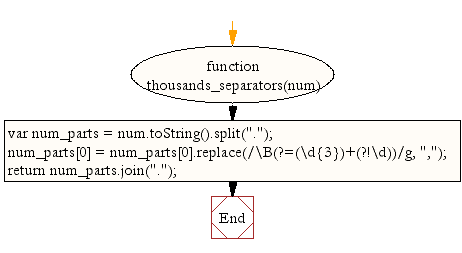
Live Demo:
See the Pen javascript-math-exercise-39 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that inserts commas as thousands separators into an integer using regular expressions.
- Write a JavaScript function that formats both integers and floating-point numbers with proper thousands grouping.
- Write a JavaScript function that manually iterates through a number's digits to insert commas at appropriate intervals.
- Write a JavaScript function that processes large numbers and ensures the decimal portion remains unaltered during formatting.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript function to check if a number is a whole number or has a decimal place.
Next: Write a JavaScript function to create random background color.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.