JavaScript: Check if a number is a whole number or has a decimal place
JavaScript Math: Exercihse-38 with Solution
Check If Number Has Decimal
Write a JavaScript function to check if a number is a whole number or has a decimal place.
Note : Whole Numbers are simply the numbers 0, 1, 2, 3, 4, 5, ... (and so on). No Fractions!
Test Data :
console.log(number_test(25.66));
"Number has a decimal place."
console.log(number_test(10));
"It is a whole number."
Visual Presentation:
Sample Solution:
JavaScript Code:
// Define a function named number_test that checks if a number has a decimal place.
function number_test(n)
{
// Calculate the difference between n and its floor value, and check if it is not equal to zero.
var result = (n - Math.floor(n)) !== 0;
// If result is true, return 'Number has a decimal place.'; otherwise, return 'It is a whole number.'
if (result)
return 'Number has a decimal place.';
else
return 'It is a whole number.';
}
// Output the result of testing if 25.66 has a decimal place to the console.
console.log(number_test(25.66));
// Output the result of testing if 10 has a decimal place to the console.
console.log(number_test(10));
Output:
Number has a decimal place. It is a whole number.
Flowchart:
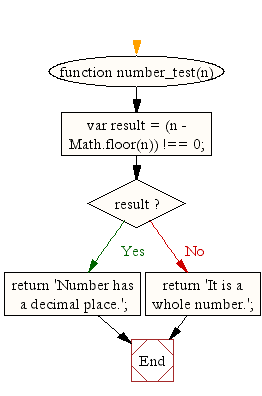
Live Demo:
See the Pen javascript-math-exercise-38 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that determines if a number is a whole number by checking for a fractional part using modulo.
- Write a JavaScript function that converts a number to a string and checks for the presence of a decimal point.
- Write a JavaScript function that compares Math.floor and Math.ceil of a number to decide if it has a decimal portion.
- Write a JavaScript function that uses bitwise operators to check if a number is integer-like or contains decimals.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript function to limit a value inside a certain range.
Next: Write a JavaScript function to print an integer with commas as thousands separators.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.