JavaScript: The Pythagorean theorem
JavaScript Math: Exercise-35 with Solution
Pythagorean Theorem Formula
Write a JavaScript function for the Pythagorean theorem.
According to Wikipedia : In mathematics, the Pythagorean theorem, also known as Pythagoras' theorem, is a relation in Euclidean geometry among the three sides of a right triangle. It states that the square of the hypotenuse (the side opposite the right angle) is equal to the sum of the squares of the other two sides. The theorem can be written as an equation relating the lengths of the sides a, b and c, often called the "Pythagorean equation".
Test Data :
console.log(pythagorean(4, 3));
5
Sample Solution:
JavaScript Code:
// Define a function named pythagorean that calculates the length of the hypotenuse of a right triangle given the lengths of the other two sides.
function pythagorean(sideA, sideB){
// Use the Pythagorean theorem to calculate the length of the hypotenuse.
return Math.sqrt(Math.pow(sideA, 2) + Math.pow(sideB, 2));
}
// Output the result of calculating the hypotenuse of a triangle with side lengths 4 and 3 to the console.
console.log(pythagorean(4, 3));
Output:
5
Visual Presentation:
Flowchart:
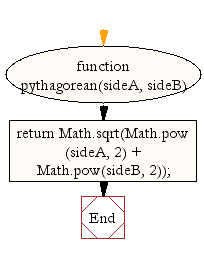
Live Demo:
See the Pen javascript-math-exercise-35 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript function to convert radians to degrees.
Next: Write a JavaScript function which will return values that are powers of two.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics