JavaScript: Convert radians to degrees
JavaScript Math: Exercise-34 with Solution
Convert Radians to Degrees
Write a JavaScript function to convert radians to degrees.
Test Data :
console.log(radians_to_degrees(0.7853981633974483));
45
Sample Solution-1:
JavaScript Code:
// Define a function named radians_to_degrees that converts radians to degrees.
function radians_to_degrees(radians)
{
// Store the value of pi.
var pi = Math.PI;
// Multiply radians by 180 divided by pi to convert to degrees.
return radians * (180/pi);
}
// Output the result of converting approximately 0.785 radians to degrees to the console.
console.log(radians_to_degrees(0.7853981633974483));
Output:
45
Visual Presentation:
Flowchart:
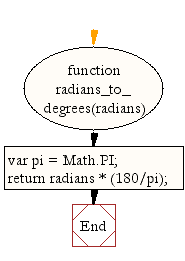
Sample Solution-2:
JavaScript Code:
// Define a function named radians_to_degrees that converts radians to degrees using an arrow function.
const radians_to_degrees = rad => (rad * 180.0) / Math.PI;
// Output the result of converting pi/2 radians to degrees to the console.
console.log(radians_to_degrees(Math.PI / 2));
// Output the result of converting approximately 0.785 radians to degrees to the console.
console.log(radians_to_degrees(0.7853981633974483));
Output:
90 45
Live Demo:
See the Pen javascript-math-exercise-34 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that converts radians to degrees using the formula (radians * 180 / Math.PI).
- Write a JavaScript function that converts an array of radian values to degrees, handling precision issues.
- Write a JavaScript function that validates a radian input and converts it to degrees, rounding to the nearest integer.
- Write a JavaScript function that performs the conversion from radians to degrees without using Math.PI directly.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript function to convert an angle from degrees to radians.
Next: Write a JavaScript function for the Pythagorean theorem.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.