JavaScript: Convert an angle from degrees to radians
JavaScript Math: Exercise-33 with Solution
Convert Degrees to Radians
Write a JavaScript function to convert an angle from degrees to radians.
Test Data :
console.log(degrees_to_radians(45));
0.7853981633974483
Use Math.PI and the degree to radian formula to convert the angle from degrees to radians.
Sample Solution-1:
JavaScript Code:
// Define a function named degrees_to_radians that converts degrees to radians.
function degrees_to_radians(degrees)
{
// Store the value of pi.
var pi = Math.PI;
// Multiply degrees by pi divided by 180 to convert to radians.
return degrees * (pi/180);
}
// Output the result of converting 45 degrees to radians to the console.
console.log(degrees_to_radians(45));
Output:
0.7853981633974483
Visual Presentation:
Flowchart:
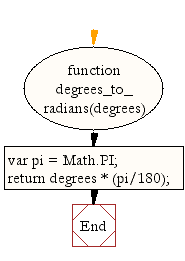
Sample Solution-2:
JavaScript Code:
/**
* Function to convert degrees to radians.
* @param {number} deg - The angle in degrees.
* @returns {number} - The angle in radians.
*/
const degrees_to_radians = deg => (deg * Math.PI) / 180.0; // Convert degrees to radians using the formula: radians = (degrees * Math.PI) / 180
console.log(degrees_to_radians(45)); // Print the result of converting 45 degrees to radians
console.log(degrees_to_radians(90)); // Print the result of converting 90 degrees to radians
Output:
0.7853981633974483 1.5707963267948966
Live Demo:
See the Pen javascript-math-exercise-33 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that converts an angle in degrees to radians using the formula (degrees * Math.PI / 180).
- Write a JavaScript function that validates the input angle and converts it to radians, rounding to a specified precision.
- Write a JavaScript function that converts an array of degree values to radians in one go.
- Write a JavaScript function that implements the conversion without directly using Math.PI by approximating its value.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript function to calculate the percentage (%) of a number.
Next: Write a JavaScript function to convert radians to degrees.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.