JavaScript: Cast a square root of a number to an integer
JavaScript Math: Exercise-30 with Solution
Cast Square Root to Integer
Write a JavaScript function to cast the square root of a number to an integer.
Test Data:
console.log(sqrt_to_int(17));
4
Sample Solution:
JavaScript Code:
// Define a function named sqrt_to_int that calculates the square root of a number and returns the integer part of the result.
function sqrt_to_int(num)
{
// Compute the square root of num using Math.sqrt(), convert it to a string, and parse it to an integer using parseInt().
return parseInt(Math.sqrt(num)+"");
}
// Output the result of calculating the square root of 17 and converting it to an integer to the console.
console.log(sqrt_to_int(17));
Output:
4
Flowchart:
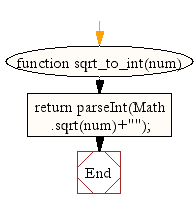
Live Demo:
See the Pen javascript-math-exercise-30 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that computes the square root of a number and casts the result to an integer by truncating decimals.
- Write a JavaScript function that calculates the square root and uses bitwise operators to extract the integer portion.
- Write a JavaScript function that returns the integer part of the square root using Math.floor, and handles edge cases.
- Write a JavaScript function that computes the square root and then casts it to an integer, ensuring proper rounding for negatives.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript function to convert a positive number to negative number.
Next: Write a JavaScript function to get the highest number from three different numbers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.