JavaScript: Convert a decimal number to binary, hexadecimal or octal number
JavaScript Math: Exercise-3 with Solution
Write a JavaScript function to convert a decimal number to a binary, hexadecimal or octal number.
Test Data:
console.log(dec_to_bho(120,'B'));
console.log(dec_to_bho(120,'H'));
console.log(dec_to_bho(120,'O'));
"1111000"
"78"
"170"
Visual Presentation:
Sample Solution:
JavaScript Code:
// Define a function named dec_to_bho that takes a decimal number (n) and a base ('B', 'H', or 'O') as input.
dec_to_bho = function(n, base) {
// If the decimal number is negative, convert it to its two's complement representation.
if (n < 0) {
n = 0xFFFFFFFF + n + 1;
}
// Switch statement to determine the base for conversion.
switch (base) {
// If base is 'B' (binary), convert decimal to binary using parseInt and toString methods.
case 'B':
return parseInt(n, 10).toString(2);
break;
// If base is 'H' (hexadecimal), convert decimal to hexadecimal using parseInt and toString methods.
case 'H':
return parseInt(n, 10).toString(16);
break;
// If base is 'O' (octal), convert decimal to octal using parseInt and toString methods.
case 'O':
return parseInt(n, 10).toString(8);
break;
// If base is not recognized, return an error message.
default:
return("Wrong input.........");
}
}
// Output the conversion of the decimal number 120 to binary, hexadecimal, and octal formats respectively.
console.log(dec_to_bho(120,'B'));
console.log(dec_to_bho(120,'H'));
console.log(dec_to_bho(120,'O'));
Output:
1111000 78 170
Explanation:
In the exercise above,
- The code defines a function called "dec_to_bho()" which takes two parameters: a decimal number 'n' and a base ('B' for binary, 'H' for hexadecimal, or 'O' for octal).
- Inside the function, there's a check to handle negative numbers. If 'n' is negative, it is converted to its two's complement representation to ensure proper conversion.
- The function then uses a "switch" statement to determine the base for conversion:
- If the base is 'B' (binary), it converts the decimal number to binary using "parseInt()" with base 10 and "toString()" with base 2.
- If the base is 'H' (hexadecimal), it converts the decimal number to hexadecimal using "parseInt()" with base 10 and "toString()" with base 16.
- If the base is 'O' (octal), it converts the decimal number to octal using "parseInt()" with base 10 and "toString()" with base 8.
- If the base is not recognized, it returns an error message.
Flowchart:
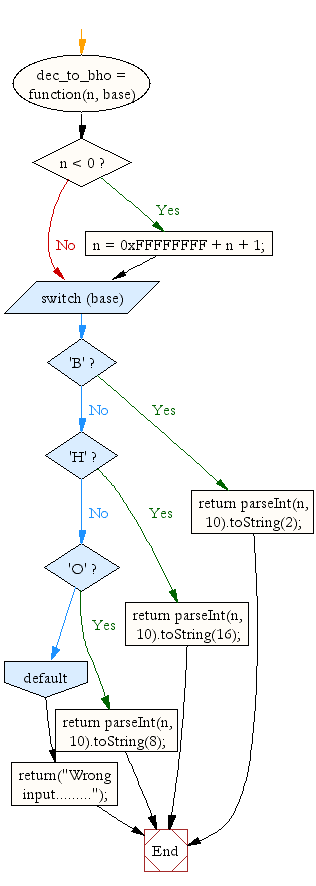
Live Demo:
See the Pen javascript-math-exercise-3 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript function to convert a binary number to a decimal number.
Next: Write a JavaScript function to generate a random integer.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics