JavaScript: Round up an integer value to the next multiple of 5
JavaScript Math: Exercise-28 with Solution
Write a JavaScript function to round up an integer value to the next multiple of 5.
Test Data:
console.log(int_round5(32));
35
console.log(int_round5(137));
140
Sample Solution:
JavaScript Code:
// Define a function named int_round5 that rounds a number up to the nearest multiple of 5.
function int_round5(num)
{
// Divide the number by 5, round the result up to the nearest integer, then multiply by 5.
return Math.ceil(num/5)*5;
}
// Output the result of rounding 32 up to the nearest multiple of 5 to the console.
console.log(int_round5(32));
// Output the result of rounding 137 up to the nearest multiple of 5 to the console.
console.log(int_round5(137));
// Output the result of rounding 142 up to the nearest multiple of 5 to the console.
console.log(int_round5(142));
Output:
35 140 145
Visual Presentation:
Flowchart:
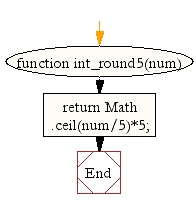
Live Demo:
See the Pen javascript-math-exercise-28 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript function to calculate degrees between 2 points with inverse Y axis.
Next: Write a JavaScript function to convert a positive number to negative number.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/javascript-exercises/javascript-math-exercise-28.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics