JavaScript: Calculate degrees between 2 points with inverse Y axis
JavaScript Math: Exercise-27 with Solution
Calculate Degrees Between Two Points
Write a JavaScript function to calculate degrees between 2 points with the inverse Y axis.
Test Data :
console.log(pointDirection(1, 0, 12, 0));
0
console.log(pointDirection(1, 0, 1, 10));
90
Sample Solution:
JavaScript Code:
// Define a function named pointDirection that calculates the direction angle from point 1 to point 2.
function pointDirection(x1, y1, x2, y2) {
// Calculate the angle using arctangent, and convert it from radians to degrees.
return Math.atan2(y2 - y1, x2 - x1) * 180 / Math.PI;
}
// Output the result of calculating the direction angle from (1, 0) to (12, 0) to the console.
console.log(pointDirection(1, 0, 12, 0));
// Output the result of calculating the direction angle from (1, 0) to (1, 10) to the console.
console.log(pointDirection(1, 0, 1, 10));
Output:
0 90
Flowchart:
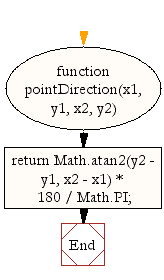
Live Demo:
See the Pen javascript-math-exercise-27 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that calculates the angle in degrees between two points in a 2D plane using inverse trigonometry.
- Write a JavaScript function that computes the directional bearing from one point to another, normalizing the angle between 0 and 360 degrees.
- Write a JavaScript function that calculates the angle between two points and accounts for the inverted Y-axis in screen coordinates.
- Write a JavaScript function that determines the angle between two coordinates and rounds the result to the nearest whole degree.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript function to calculate the nth root of a number.
Next: Write a JavaScript function to round up an integer value to the next multiple of 5.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics