JavaScript: Calculate the nth root of a number
JavaScript Math: Exercise-26 with Solution
Write a JavaScript function to calculate the nth root of a number.
Test Data :
console.log(nthroot(64, 2));
8
console.log(nthroot(64, -2));
0.125
Sample Solution:
JavaScript Code:
// Define a function named nthroot that calculates the nth root of a given number.
function nthroot(x, n)
{
// Determine if n is odd.
ng = n % 2;
// If n is odd or x is negative, make x positive.
if((ng == 1) || x<0)
x = -x;
// Calculate the nth root of x.
var r = Math.pow(x, 1 / n);
// Raise r to the power of n.
n = Math.pow(r, n);
// Check if the difference between x and n is less than 1 and both x and n have the same sign.
if(Math.abs(x - n) < 1 && (x > 0 === n > 0))
// If n is odd, return -r, otherwise return r.
return ng ? -r : r;
}
// Output the result of calculating the square root of 64 to the console.
console.log(nthroot(64, 2));
// Output the result of calculating the square root of 64 with negative exponent to the console.
console.log(nthroot(64, -2));
Output:
8 0.125
Visual Presentation:
Flowchart:
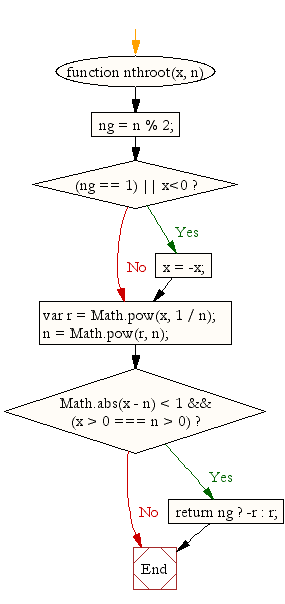
Live Demo:
See the Pen javascript-math-exercise-26 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript function to make currency math (add, subtract, multiply, division etc.).
Next: Write a JavaScript function to calculate degrees between 2 points with inverse Y axis.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics