JavaScript: Function to create a UUID identifier
JavaScript Math: Exercise-23 with Solution
Generate UUID Identifier
Write a JavaScript function to create a UUID identifier.
Note :
According to Wikipedia - A universally unique identifier (UUID) is an identifier standard used in software construction. A UUID is simply a 128-bit value. The meaning of each bit is defined by any of several variants. For human-readable display, many systems use a canonical format using hexadecimal text with inserted hyphen characters. For example : de305d54-75b4-431b-adb2-eb6b9e546014
Sample Solution:
JavaScript Code:
// Define a function named create_UUID that generates a version 4 UUID.
function create_UUID(){
// Get the current time in milliseconds since the Unix epoch.
var dt = new Date().getTime();
// Replace the placeholders in the UUID template with random hexadecimal characters.
var uuid = 'xxxxxxxx-xxxx-4xxx-yxxx-xxxxxxxxxxxx'.replace(/[xy]/g, function(c) {
// Generate a random hexadecimal digit.
var r = (dt + Math.random()*16)%16 | 0;
// Update dt to simulate passage of time for the next random character.
dt = Math.floor(dt/16);
// Replace 'x' with a random digit and 'y' with a specific digit (4 for UUID version 4).
return (c=='x' ? r :(r&0x3|0x8)).toString(16);
});
// Return the generated UUID.
return uuid;
}
// Output a version 4 UUID to the console.
console.log(create_UUID());
Output:
1fe35579-5ce7-46ec-89e0-7e7236700297
Flowchart:
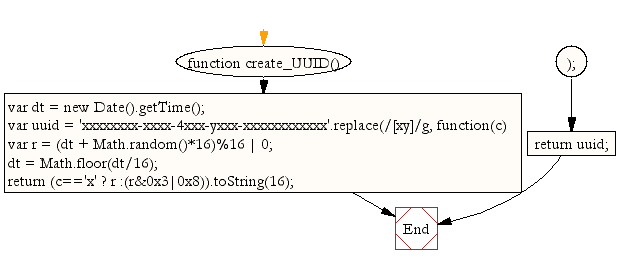
Live Demo:
See the Pen javascript-math-exercise-23 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that generates a random UUID (version 4) using random hexadecimal digits and proper hyphenation.
- Write a JavaScript function that creates a UUID and validates its format using a regular expression.
- Write a JavaScript function that simulates UUID generation by combining the current timestamp with random numbers.
- Write a JavaScript function that generates an array of UUIDs ensuring each one is unique and correctly formatted.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript function that Convert Roman Numeral to Integer.
Next: Write a JavaScript function to round a number to a specified number of digits and strip extra zeros (if any).
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.