JavaScript: Convert an integer into a Roman Numeral
JavaScript Math: Exercise-21 with Solution
Integer to Roman Numeral Conversion
Write a JavaScript function that converts an integer into a Roman numeral.
Sample Solution:
Visual Presentation:
JavaScript Code:
// Define a function named integer_to_roman that converts an integer to its Roman numeral representation.
function integer_to_roman(num) {
// Check if num is not a number, if so, return false.
if (typeof num !== 'number')
return false;
// Split the digits of num and store them in an array.
var digits = String(+num).split(""),
// Define an array representing Roman numeral symbols for different place values.
key = ["","C","CC","CCC","CD","D","DC","DCC","DCCC","CM",
"","X","XX","XXX","XL","L","LX","LXX","LXXX","XC",
"","I","II","III","IV","V","VI","VII","VIII","IX"],
// Initialize an empty string to store the Roman numeral.
roman_num = "",
// Set a counter to determine the place value (ones, tens, hundreds, etc.).
i = 3;
// Iterate through the digits array to construct the Roman numeral representation.
while (i--)
roman_num = (key[+digits.pop() + (i * 10)] || "") + roman_num;
// Add 'M' symbols for the thousands place.
return Array(+digits.join("") + 1).join("M") + roman_num;
}
// Output the Roman numeral representation of the integer 27 to the console.
console.log(integer_to_roman(27));
Output:
XXVII
Flowchart:
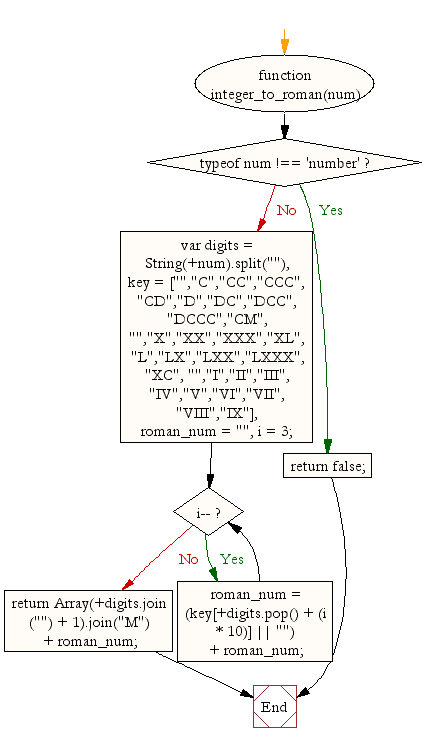
Live Demo:
See the Pen javascript-math-exercise-21 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that converts an integer to a Roman numeral using iterative subtraction with mapping.
- Write a JavaScript function that recursively converts an integer to its Roman numeral representation.
- Write a JavaScript function that implements a mapping of decimal values to Roman symbols and assembles the numeral accordingly.
- Write a JavaScript function that converts an integer to a Roman numeral and validates that the number is within 1 to 3999.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript program to evaluate binomial coefficients.
Next: Write a JavaScript function that Convert Roman Numeral to Integer.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.