JavaScript: Evaluate binomial coefficients
JavaScript Math: Exercise-20 with Solution
Binomial Coefficients
Write a JavaScript program to evaluate binomial coefficients.
Note:
Binomial coefficient : According to Wikipedia - In mathematics, binomial coefficients are a family of positive integers that occur as coefficients in the binomial theorem. They are indexed by two nonnegative integers; the binomial coefficient indexed by n and k is usually written \tbinom nk. It is the coefficient of the x k term in the polynomial expansion of the binomial power (1 + x) n. Under suitable circumstances the value of the coefficient is given by the expression :

Arranging binomial coefficients into rows for successive values of n, and in which k ranges from 0 to n, gives a triangular array called Pascal's triangle.
Test Data:
console.log(binomial(8,3));
console.log(binomial(10,2));
Output :
56
45
Sample Solution:
JavaScript Code:
// Define a function named binomial that calculates the binomial coefficient "n choose k".
function binomial(n, k) {
// Check if both n and k are of type number, if not, return false.
if ((typeof n !== 'number') || (typeof k !== 'number'))
return false;
// Initialize the coefficient variable to 1.
var coeff = 1;
// Calculate the numerator of the binomial coefficient.
for (var x = n - k + 1; x <= n; x++) coeff *= x;
// Calculate the denominator of the binomial coefficient.
for (x = 1; x <= k; x++) coeff /= x;
// Return the calculated binomial coefficient.
return coeff;
}
// Output the result of calculating the binomial coefficient "8 choose 3" to the console.
console.log(binomial(8, 3));
// Output the result of calculating the binomial coefficient "10 choose 2" to the console.
console.log(binomial(10, 2));
Output:
56 45
Flowchart:
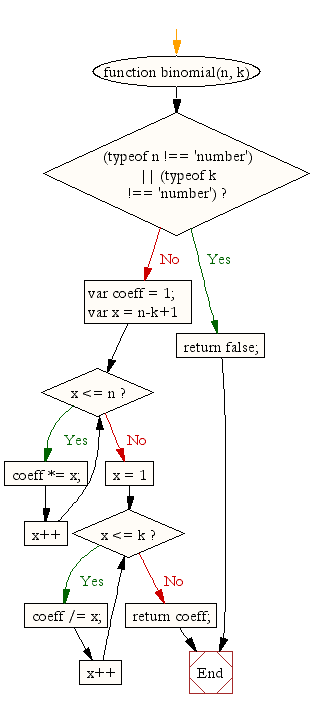
Live Demo:
See the Pen javascript-math-exercise-20 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that calculates the binomial coefficient recursively with memoization.
- Write a JavaScript function that computes binomial coefficients iteratively using dynamic programming.
- Write a JavaScript function that returns the nth row of Pascal's triangle by computing binomial coefficients.
- Write a JavaScript function that validates non-negative integer inputs before computing the binomial coefficient.
Improve this sample solution and post your code through Disqus.
Previous: Create a Pythagorean function in JavaScript.
Next: Write a JavaScript function that Convert an integer into a Roman numeral.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.