JavaScript : Pythagorean function in JavaScript
JavaScript Math: Exercise-19 with Solution
Create a Pythagorean function in JavaScript.
Note : The Pythagorean Theorem tells us that the relationship in every right triangle is : c2 = a2 + b2, where c is the hypotenuse and a, b are two legs of the triangle.
Test Data:
console.log(pythagorean_theorem(2, 4));
console.log(pythagorean_theorem(3, 4));
Output :
4.47213595499958
5
Sample Solution:
JavaScript Code:
// Define a function named pythagorean_theorem that calculates the hypotenuse of a right triangle given the lengths of its two legs.
function pythagorean_theorem(x, y) {
// Check if both x and y are of type number, if not, return false.
if ((typeof x !== 'number') || (typeof y !== 'number'))
return false;
// Calculate and return the square root of the sum of the squares of x and y (hypotenuse).
return Math.sqrt(x * x + y * y);
}
// Output the result of calculating the hypotenuse of a triangle with legs of lengths 2 and 4 to the console.
console.log(pythagorean_theorem(2, 4));
// Output the result of calculating the hypotenuse of a triangle with legs of lengths 3 and 4 to the console.
console.log(pythagorean_theorem(3, 4));
Output:
4.47213595499958 5
Flowchart:
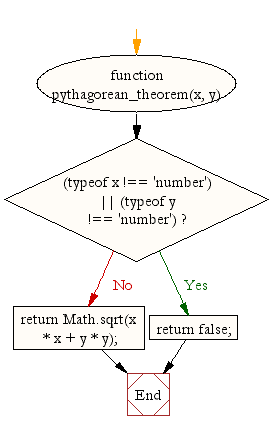
Live Demo:
See the Pen javascript-math-exercise-19 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript function to calculate the product of values in an array.
Next: Write a JavaScript program to evaluate binomial coefficients.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/javascript-exercises/javascript-math-exercise-19.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics