JavaScript: Check whether a value is an integer or not
JavaScript Math: Exercise-15 with Solution
Check If Value is Integer
Write a JavaScript function to check whether a value is an integer or not.
Test Data:
console.log(is_Int(23));
console.log(is_Int(4e2));
console.log(is_Int(NaN));
console.log(is_Int(23.75));
console.log(is_Int(-23));
Output :
true
true
false
false
true
Visual Presentation:
Sample Solution:
JavaScript Code:
// Define a function named is_Int that checks if a number is an integer.
function is_Int(num) {
// Check if the input is not a number, if so, return false.
if (typeof num !== 'number')
return false;
// Check if the number is not NaN, if its integer representation equals itself, and if parsing it as an integer with base 10 does not result in NaN.
return !isNaN(num) &&
parseInt(Number(num)) == num &&
!isNaN(parseInt(num, 10));
}
// Output the result of checking if 23 is an integer to the console.
console.log(is_Int(23));
// Output the result of checking if 4e2 is an integer to the console.
console.log(is_Int(4e2));
// Output the result of checking if NaN is an integer to the console.
console.log(is_Int(NaN));
// Output the result of checking if 23.75 is an integer to the console.
console.log(is_Int(23.75));
// Output the result of checking if -23 is an integer to the console.
console.log(is_Int(-23));
Output:
true true false false true
Flowchart:
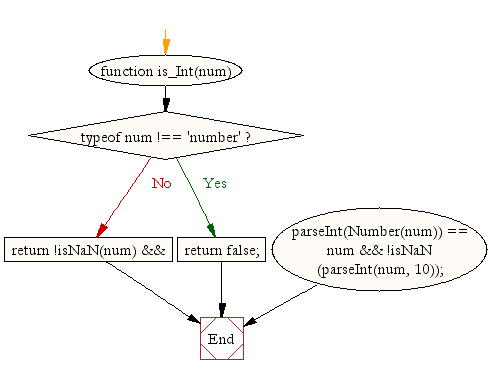
Live Demo:
See the Pen javascript-math-exercise-15 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that checks if a value is an integer using Number.isInteger and fallback logic for older browsers.
- Write a JavaScript function that determines integer status by comparing Math.floor and Math.ceil of the value.
- Write a JavaScript function that tests if a value is an integer after converting numeric strings, handling edge cases.
- Write a JavaScript function that uses bitwise operations to check if a value is an integer and returns a boolean.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript function to round a number to a given decimal places.
Next: Write a JavaScript function to check whether a variable is numeric or not.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.