JavaScript: Test if a number is a power of 2
JavaScript Math: Exercise-13 with Solution
Check Power of 2
Write a JavaScript function to test if a number is a power of 2.
Test Data:
console.log(power_of_2(16));
console.log(power_of_2(18));
console.log(power_of_2(256));
Output:
true
false
true
Visual Presentation:
Sample Solution-1:
JavaScript Code:
// Define a constant named power_of_2 using arrow function syntax that checks if a number is a power of 2.
const power_of_2 = n => !!n && (n & (n - 1)) == 0;
// Output the result of checking if 16 is a power of 2 to the console.
console.log(power_of_2(16));
// Output the result of checking if 18 is a power of 2 to the console.
console.log(power_of_2(18));
// Output the result of checking if 256 is a power of 2 to the console.
console.log(power_of_2(256));
Output:
true false true
Flowchart:
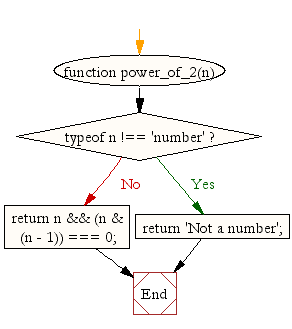
Sample Solution-2:
JavaScript Code:
const power_of_2 = n => !!n && (n & (n - 1)) == 0;
console.log(power_of_2(16));
console.log(power_of_2(18));
console.log(power_of_2(256));
Sample Output:
true false true
Live Demo:
See the Pen javascript-math-exercise-13 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that checks if a number is a power of 2 using bitwise operations.
- Write a JavaScript function that recursively divides a number by 2 to determine if it is a power of 2.
- Write a JavaScript function that converts a number to binary and checks if it contains only one '1' bit.
- Write a JavaScript function that uses logarithms to test if a number's logarithm (base 2) is an integer.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript function to find out if a number is a natural number or not.
Next: Write a JavaScript function to round a number to a given decimal places.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.