JavaScript: Smallest number whose digits multiply to a number
JavaScript Math: Exercise-113 with Solution
Smallest Number from Digit Product
Write a JavaScript program to calculate the smallest number whose digits multiply into a given number.
Example:
let n =100
Test Data:
(100) -> 455
(120) -> 358
(16) -> 28
(129) -> 0
Sample Solution:
JavaScript Code:
/**
* Function to find the smallest number whose digits multiply to the given number.
* @param {number} n - The given number.
* @returns {number} - The smallest number whose digits multiply to the given number.
*/
function test(n) {
if (n < 10) return n; // If n is less than 10, return n itself
data = []; // Array to store all occurrences of digits
for (i = 9; i > 1; i--) {
// Store all occurrences of current digit in data
// if current digit divides n.
while (n % i === 0) {
n = n / i;
data.push(i);
}
}
if (n != 1) return 0; // If n is not 1, it means it contains a prime number greater than 9, so return 0
result = 0; // Initialize result to store the smallest number whose digits multiply to n
for (i = data.length - 1; i >= 0; i--) {
result = result * 10 + data[i]; // Build the result number from the array data
if (result > Number.MAX_VALUE) return 0; // If result exceeds the maximum value for a number, return 0
}
return result; // Return the smallest number whose digits multiply to n
}
// Test cases
n = 100;
console.log("n = " + n);
console.log("Smallest number whose digits multiply to the said number: " + test(n));
n = 120;
console.log("n = " + n);
console.log("Smallest number whose digits multiply to the said number: " + test(n));
n = 16;
console.log("n = " + n);
console.log("Smallest number whose digits multiply to the said number: " + test(n));
n = 129;
console.log("n = " + n);
console.log("Smallest number whose digits multiply to the said number: " + test(n));
Output:
n = 100 Smallest number whose digits multiply to the said number: 455 n = 120 Smallest number whose digits multiply to the said number: 358 n = 16 Smallest number whose digits multiply to the said number: 28 n = 129 Smallest number whose digits multiply to the said number: 0
Flowchart:
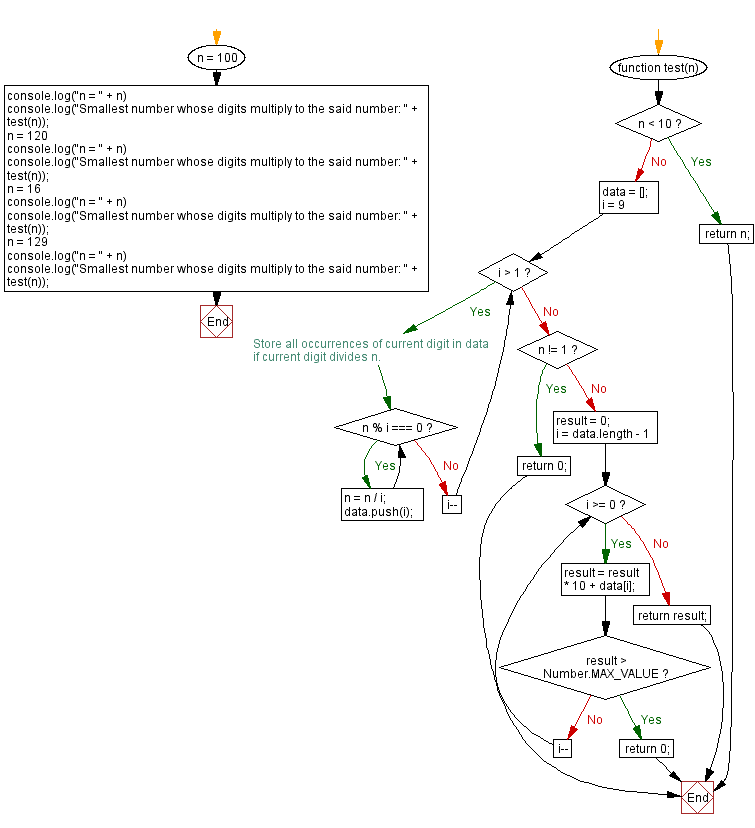
Live Demo:
See the Pen javascript-math-exercise-113 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that finds the smallest number whose digits multiply to a given number by factorizing the input.
- Write a JavaScript function that decomposes a number into factors and arranges them to form the smallest possible integer.
- Write a JavaScript function that handles cases where the digit product cannot form a valid number and returns an error.
- Write a JavaScript function that uses a greedy algorithm to construct the minimal number from the factors of the input product.
Improve this sample solution and post your code through Disqus.
Previous: Maximum value swapping two digits in an integer.
Next: Check a given number is self-dividing or not.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.