JavaScript: Maximum value swapping two digits in an integer
JavaScript Math: Exercise-112 with Solution
Max Value by Digit Swap
Write a JavaScript program to calculate the maximum value by swapping two digits in a given integer.
Test Data:
(100) -> 100
(120) -> 210
(129) -> 921
Sample Solution:
JavaScript Code:
/**
* Function to find the maximum value by swapping two digits in the given integer.
* @param {number} n - The integer.
* @returns {number} - The maximum value obtained after swapping two digits, if any.
*/
function test(n) {
// Convert the integer to a string
var n_str = n.toString();
// Loop through each digit of the integer
for (var i = 0; i < n_str.length; i++) {
var t = n_str[i]; // Current digit
var index = i; // Index of the current digit
// Find the maximum digit greater than the current digit
for (var x = n_str.length - 1; x > i; x--) {
if (n_str[x] > t) {
t = n_str[x];
index = x;
}
}
// If a greater digit is found, swap the digits and return the new integer
if (t != n_str[i]) {
var nums = n_str.split('');
var tmp = nums[i];
nums[i] = nums[index];
nums[index] = tmp;
return parseInt(nums.join(''));
}
}
// If no swap is possible, return the original integer
return n;
}
// Test cases
n = 100;
console.log("n = " + n);
console.log("Maximum value swapping two digits in the said integer: " + test(n));
n = 120;
console.log("n = " + n);
console.log("Maximum value swapping two digits in the said integer: " + test(n));
n = 129;
console.log("Maximum value swapping two digits in the said integer: " + test(n));
console.log("e: " + test(n));
Output:
n = 100 Maximum value swapping two digits in the said integer: 100 n = 120 Maximum value swapping two digits in the said integer: 210 Maximum value swapping two digits in the said integer: 921 e: 921
Flowchart:
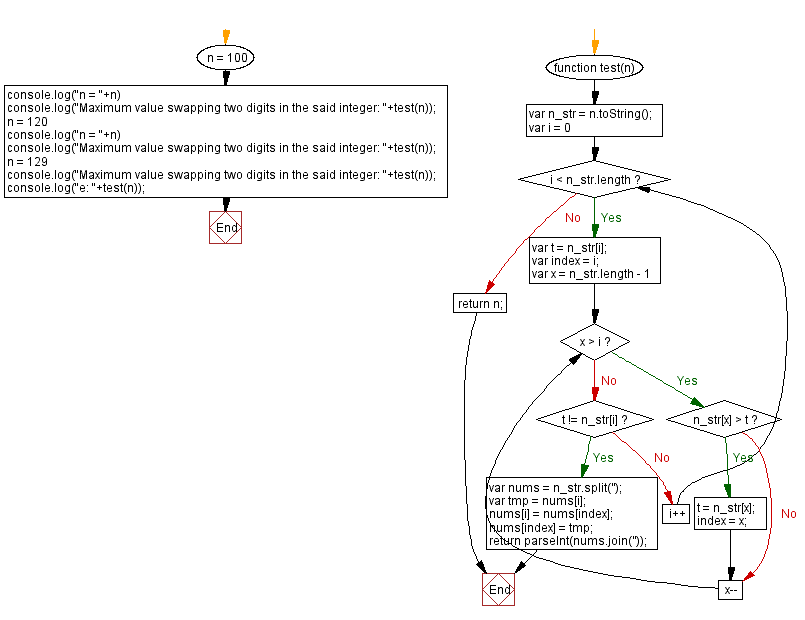
Live Demo:
See the Pen javascript-math-exercise-112 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that finds the maximum possible value by swapping two digits in a given number.
- Write a JavaScript function that converts a number to a string, explores all single-swap possibilities, and returns the maximum value.
- Write a JavaScript function that iterates over digit pairs to determine the best swap that results in the highest number.
- Write a JavaScript function that handles edge cases such as numbers with identical digits and returns the original number if no beneficial swap exists.
Improve this sample solution and post your code through Disqus.
Previous: Kth smallest number in a multiplication table.
Next: Smallest number whose digits multiply to a number.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.