JavaScript: Least common multiple (LCM) of more than 2 integers
JavaScript Math: Exercise-11 with Solution
LCM of Multiple Numbers
Write a JavaScript function to get the least common multiple (LCM) of more than 2 integers.
Test Data :
console.log(lcm_more_than_two_numbers([100,90,80,7]));
console.log(lcm_more_than_two_numbers([5,10,15,25]));
Output :
25200
150
Sample Solution:
JavaScript Code:
// Define a function named lcm_more_than_two_numbers that calculates the least common multiple (LCM) of an array of numbers.
function lcm_more_than_two_numbers(input_array) {
// Check if the input is an array, if not, return false.
if (toString.call(input_array) !== "[object Array]")
return false;
var r1 = 0, r2 = 0;
// Get the length of the input array.
var l = input_array.length;
// Iterate through the input array to calculate the LCM.
for(i = 0; i < l; i++) {
// Calculate the remainder when dividing the current element by the next element.
r1 = input_array[i] % input_array[i + 1];
// If the remainder is 0, update the next element to the product of the current element and the next element divided by the next element.
if (r1 === 0) {
input_array[i + 1] = (input_array[i] * input_array[i + 1]) / input_array[i + 1];
}
// If the remainder is not 0, calculate the remainder when dividing the next element by the previous remainder.
else {
r2 = input_array[i + 1] % r1;
// If the new remainder is 0, update the next element to the product of the current element and the next element divided by the previous remainder.
if (r2 === 0) {
input_array[i + 1] = (input_array[i] * input_array[i + 1]) / r1;
}
// If the new remainder is not 0, update the next element to the product of the current element and the next element divided by the new remainder.
else {
input_array[i + 1] = (input_array[i] * input_array[i + 1]) / r2;
}
}
}
// Return the last element of the modified input array, which represents the LCM.
return input_array[l - 1];
}
// Output the LCM of the numbers 100, 90, 80, and 7 to the console.
console.log(lcm_more_than_two_numbers([100, 90, 80, 7]));
// Output the LCM of the numbers 5, 10, 15, and 25 to the console.
console.log(lcm_more_than_two_numbers([5, 10, 15, 25]));
Output:
25200 150
Flowchart:
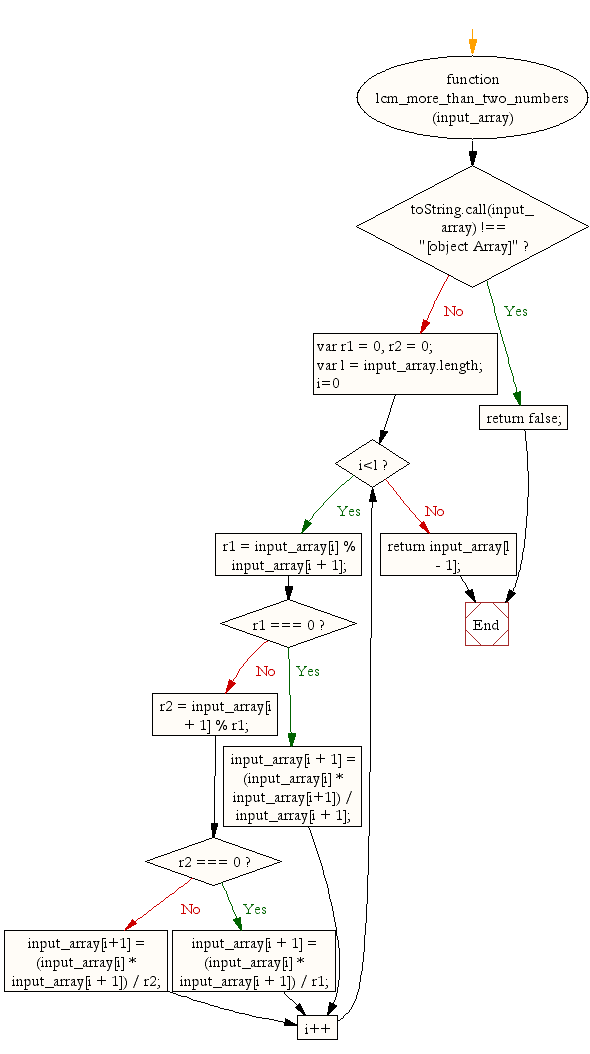
Live Demo:
See the Pen javascript-math-exercise-11 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript function to find out if a number is a natural number or not.
Next: Write a JavaScript function to get the least common multiple (LCM) of more than 2 integers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics