JavaScript - Count all the numbers with unique digits in a range
JavaScript Math: Exercise-109 with Solution
Unique Digit Numbers Count
Write a JavaScript program that accepts a number (n) and counts all numbers with unique digits of length p within a specified range.
Range: 0 <= p < 10n
Example:
When n = 1, numbers with unique digits (10) between 0 and 9 are 0, 1, 2, 3, 4, 5, 6, 7, 8, 9
When n = 2, numbers with unique digits (91) between 0 and 100 are 0,1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 12, 13, 14, 15 …..99 except 11, 22, 33, 44, 55, 66, 77, 88 and 99.
Test Data:
(1) -> 10
(2) -> 91
Sample Solution:
JavaScript Code:
/**
* Function to calculate the count of numbers with unique digits in a given range.
* @param {number} n - The upper limit of the range (from 0 to n).
* @returns {number} - The count of numbers with unique digits in the specified range.
*/
function test(n) {
// If n is 0, there's one number with a unique digit: 0 itself
if(n===0){
return 1;
}
// Initialize result to 10, as numbers from 0 to 9 all have unique digits
var result = 10;
// Initialize temp to 9, representing the number of choices for the first digit (1 to 9)
var temp = 9;
// Loop through the range starting from 1 (since we've already considered the case when n=0)
for(var i=1; i<n; i++){
// Update temp by multiplying with the number of remaining choices
temp *= 10 - i;
// Add temp to result, representing the count of numbers with unique digits at each iteration
result += temp;
}
// Return the final count of numbers with unique digits in the range
return result;
}
// Test cases
n = 1;
console.log("Range: " + n + " to 10");
console.log("Numbers with unique digits in the said range: " + test(n));
n = 2;
console.log("Range: " + n + " to 10");
console.log("Numbers with unique digits in the said range: " + test(n));
Output:
Range: 1 to 10 Numbers with unique digits in the said range: 10 Range: 2 to 10 Numbers with unique digits in the said range: 91
Flowchart:
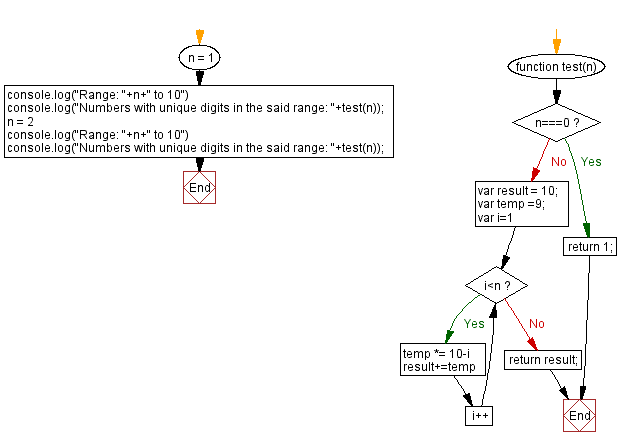
Live Demo:
See the Pen javascript-math-exercise-109 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus.
Previous: Power of four.
Next: Sum of two square numbers equal to an integer.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics