JavaScript: Power of three
JavaScript Math: Exercise-107 with Solution
Check Power of Three
Write a JavaScript program to check whether a given integer is a power of three or not.
In mathematics, a power of three is a number of the form 3n where n is an integer – that is, the result of exponentiation with number three as the base and integer n as the exponent.
Test Data:
(27) -> true
(9) -> true
(36) -> false
Sample Solution:
Solution-1
JavaScript Code:
/**
* Function to check if a number is a power of three.
* @param {number} n - The number to be checked.
* @returns {boolean} - True if the number is a power of three, false otherwise.
*/
function test(n) {
// Check if the logarithm of the number to the base 3 is an integer
return (Math.log10(n) / Math.log10(3)) % 1 == 0;
}
// Test cases
n = 27;
console.log("Number n = " + n);
console.log("Check if the said number is a power of three: " + test(n));
n = 9;
console.log("Number n = " + n);
console.log("Check if the said number is a power of three: " + test(n));
n = 36;
console.log("Number n = " + n);
console.log("Check if the said number is a power of three: " + test(n));
Output:
Number n = 27 Check the said number is power of three or not? true Number n = 9 Check the said number is power of three or not? true Number n = 36 Check the said number is power of three or not? false
Flowchart:
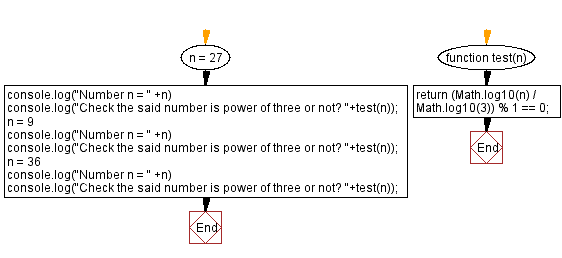
Live Demo:
See the Pen javascript-math-exercise-107 by w3resource (@w3resource) on CodePen.
Solution-2
JavaScript Code:
/**
* Function to check if a number is a power of four.
* @param {number} n - The number to be checked.
* @returns {boolean} - True if the number is a power of four, false otherwise.
*/
function test(n) {
// Check if the number is less than 1
if (n < 1) {
return false;
}
// Divide the number by 4 until it is not divisible by 4
while (n % 4 === 0) {
n = n / 4;
}
// Check if the resulting number is 1
return n === 1;
}
// Test cases
n = 16;
console.log("Number n = " + n);
console.log("Check if the said number is a power of four: " + test(n));
n = 4096;
console.log("Number n = " + n);
console.log("Check if the said number is a power of four: " + test(n));
n = 36;
console.log("Number n = " + n);
console.log("Check if the said number is a power of four: " + test(n));
Output:
Number n = 16 Check if the said number is a power of four: true Number n = 4096 Check if the said number is a power of four: true Number n = 36 Check if the said number is a power of four: false
Flowchart:
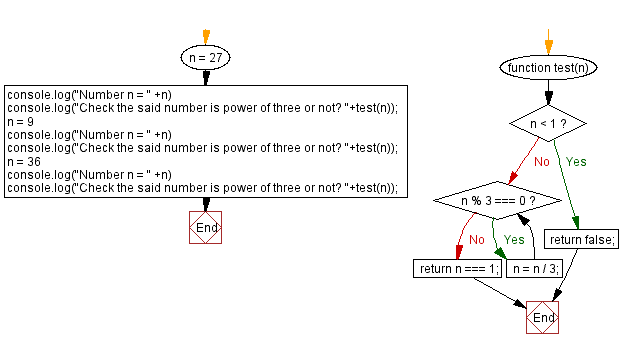
Live Demo:
See the Pen javascript-math-exercise-107-1 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that checks whether a number is a power of three by repeatedly dividing by 3.
- Write a JavaScript function that uses logarithms to determine if the base-3 logarithm of a number is an integer.
- Write a JavaScript function that recursively divides a number by three and checks if it eventually equals one.
- Write a JavaScript function that validates the input and properly handles edge cases when checking for a power of three.
Improve this sample solution and post your code through Disqus.
Previous: Least number of perfect square that sums upto n.
Next: Power of four.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.