JavaScript: Conversion of integers to English words
JavaScript Math: Exercise-105 with Solution
Number to English Representation
Write a JavaScript program that converts a non-negative integer number to its English representation.
Test Data:
(1002) -> One Thousand Two.
(1279) -> One Thousand Two Hundred Seventy Nine.
(127900) -> One Hundred Twenty Seven Thousand Nine Hundred.
(1279000) -> One Million Two Hundred Seventy Nine Thousand.
Sample Solution:
JavaScript Code:
/**
* Function to convert a given number into words.
* @param {number} n - The number to be converted into words.
* @returns {string} - The word representation of the given number.
*/
function test(n) {
if (n < 0)
return false;
// Arrays to hold words for single-digit, double-digit, and below-hundred numbers
single_digit = ['', 'One', 'Two', 'Three', 'Four', 'Five', 'Six', 'Seven', 'Eight', 'Nine']
double_digit = ['Ten', 'Eleven', 'Twelve', 'Thirteen', 'Fourteen', 'Fifteen', 'Sixteen', 'Seventeen', 'Eighteen', 'Nineteen']
below_hundred = ['Twenty', 'Thirty', 'Forty', 'Fifty', 'Sixty', 'Seventy', 'Eighty', 'Ninety']
if (n === 0) return 'Zero';
// Recursive function to translate the number into words
function translate(n) {
let word = "";
if (n < 10) {
word = single_digit[n] + ' ';
} else if (n < 20) {
word = double_digit[n - 10] + ' ';
} else if (n < 100) {
let rem = translate(n % 10);
word = below_hundred[(n - n % 10) / 10 - 2] + ' ' + rem;
} else if (n < 1000) {
word = single_digit[Math.trunc(n / 100)] + ' Hundred ' + translate(n % 100);
} else if (n < 1000000) {
word = translate(parseInt(n / 1000)).trim() + ' Thousand ' + translate(n % 1000);
} else if (n < 1000000000) {
word = translate(parseInt(n / 1000000)).trim() + ' Million ' + translate(n % 1000000);
} else {
word = translate(parseInt(n / 1000000000)).trim() + ' Billion ' + translate(n % 1000000000);
}
return word;
}
// Get the result by translating the given number
let result = translate(n);
return result.trim() + '.';
}
n = 1002;
console.log("Number n = " + n);
console.log("In word: " + test(n));
n = 1279;
console.log("Number n = " + n);
console.log("In word: " + test(n));
n = 127900;
console.log("Number n = " + n);
console.log("In word: " + test(n));
n = 1279000;
console.log("Number n = " + n);
console.log("In word: " + test(n));
Output:
Number n = 1002 In word: One Thousand Two. Number n = 1279 In word: One Thousand Two Hundred Seventy Nine. Number n = 127900 In word: One Hundred Twenty Seven Thousand Nine Hundred. Number n = 1279000 In word: One Million Two Hundred Seventy Nine Thousand.
Flowchart:
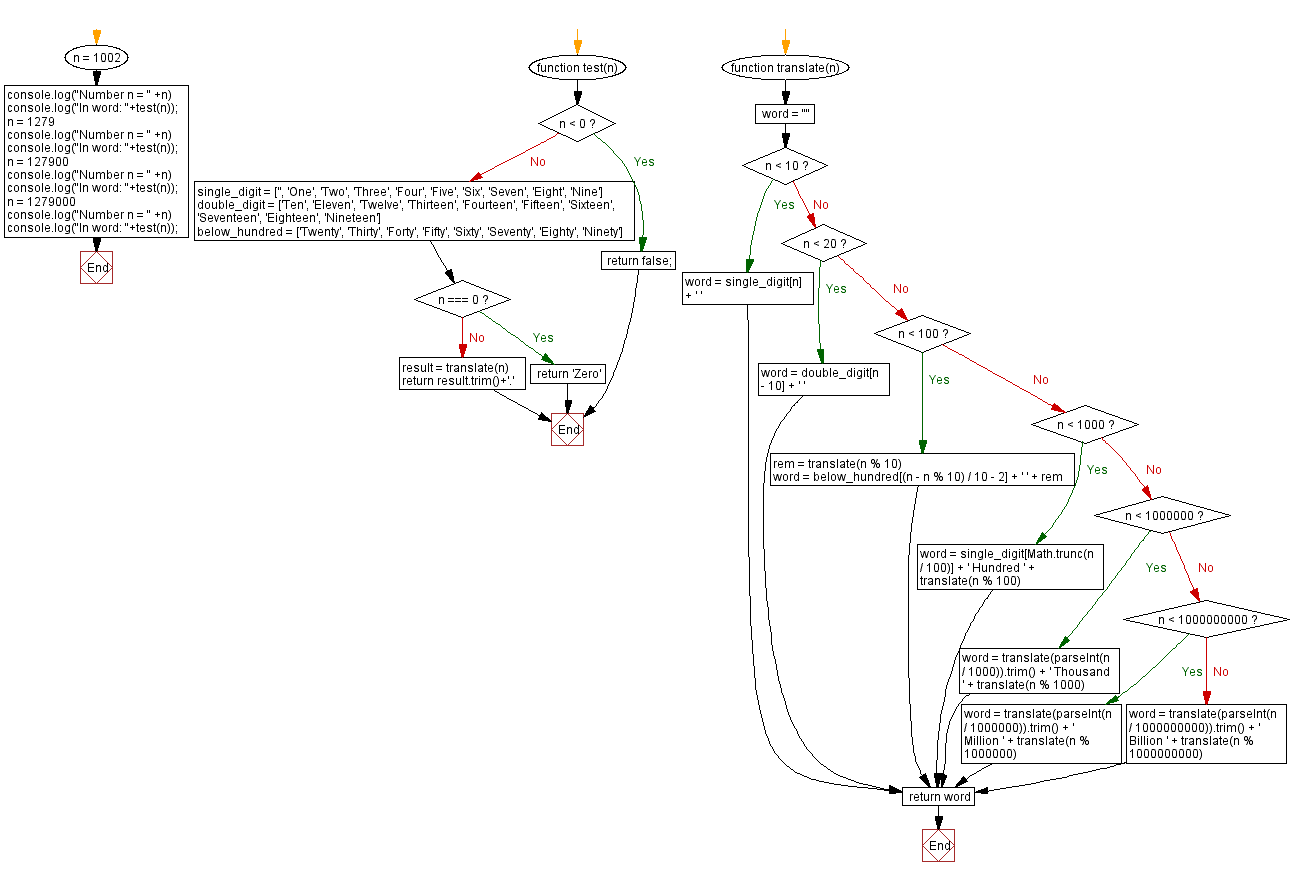
Live Demo:
See the Pen javascript-math-exercise-105 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that converts a non-negative integer to its English words representation using recursive breakdown.
- Write a JavaScript function that splits the number into segments (thousands, hundreds, tens) and converts each to words.
- Write a JavaScript function that handles special cases like zero and numbers in the teens while converting to English.
- Write a JavaScript function that formats the final English representation with proper spacing and punctuation.
Improve this sample solution and post your code through Disqus.
Previous: Distinct ways to climb the staircase.
Next: Least number of perfect square that sums upto n.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.