JavaScript: Distinct ways to climb the staircase
JavaScript Math: Exercise-104 with Solution
A staircase consists of N steps, and you are given the choice of climbing one step at a time or two steps at a time.
Write a JavaScript program to find distinct ways to climb the staircase.
Example:
Number of stairs = 2
There are 2 ways to climb the stairs: (1,1) and (2)
Number of stairs = 3
There are 3 ways to climb the stairs: (1,1,1), (2,1) and (1,2)
Visual Presentation:
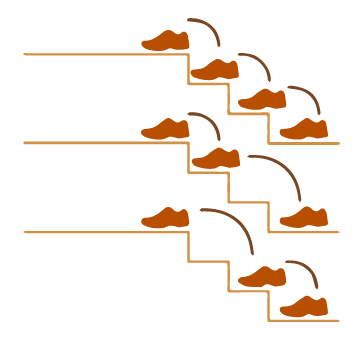
Test Data:
(2) -> 2
(3) -> 3
Sample Solution:
Solution-1
JavaScript Code:
/**
* Function to calculate the number of ways to climb a staircase with n steps.
* @param {number} n - The number of steps in the staircase.
* @returns {number} - The number of ways to climb the staircase.
*/
function test(n) {
var x = 0, // Variable to store the previous Fibonacci number
y = 1; // Variable to store the current Fibonacci number
// Loop to calculate the Fibonacci number iteratively
while (n-- > 0) {
y = x + y; // Calculate the next Fibonacci number
x = y - x; // Update the previous Fibonacci number
}
return y; // Return the current Fibonacci number
}
n = 2;
console.log("Number of stairs = " + n);
console.log("Number of ways to climb the said number of stairs: " + test(n));
n = 3;
console.log("Number of stairs = " + n);
console.log("Number of ways to climb the said number of stairs: " + test(n));
Output:
Number of stairs = 2
Flowchart:
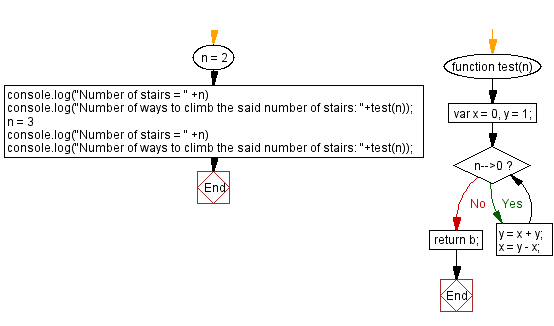
Live Demo:
See the Pen javascript-math-exercise-104 by w3resource (@w3resource) on CodePen.
Solution-2
JavaScript Code:
/**
* Function to calculate the number of ways to climb a staircase with n steps using dynamic programming.
* @param {number} n - The number of steps in the staircase.
* @returns {number} - The number of ways to climb the staircase.
*/
function test(n) {
nums = []; // Array to store the number of ways to climb each step
nums[1] = 1; // Number of ways to climb 1 step
nums[2] = 2; // Number of ways to climb 2 steps
// Loop to calculate the number of ways to climb each step from 3 to n
for (i = 3; i <= n; i++) {
nums[i] = nums[i - 1] + nums[i - 2]; // Number of ways to climb the current step
}
return nums[n]; // Return the number of ways to climb the nth step
}
n = 2;
console.log("Number of stairs = " + n);
console.log("Number of ways to climb the said number of stairs: " + test(n));
n = 3;
console.log("Number of stairs = " + n);
console.log("Number of ways to climb the said number of stairs: " + test(n));
Output:
Number of stairs = 2 Number of ways to climb the said number of stairs: 2 Number of stairs = 3 Number of ways to climb the said number of stairs: 3
Flowchart:
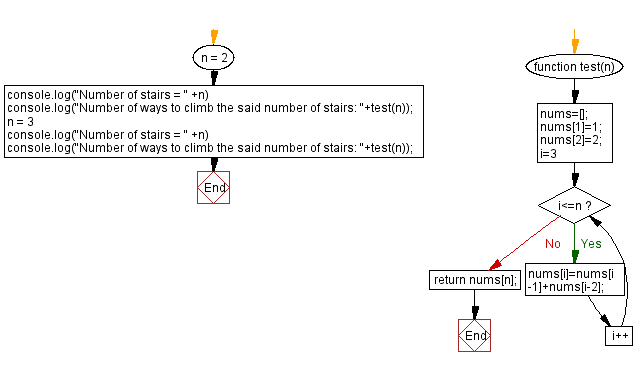
Live Demo:
See the Pen javascript-math-exercise-104-1 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus.
Previous: Product of two non-negative integers as strings.
Next: Javascript Array Exercises
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics