JavaScript: Product of two non-negative integers as strings
JavaScript Math: Exercise-103 with Solution
String Multiplication
Write a JavaScript program to calculate the product of non-negative integers n1 and n2 represented as strings. The product is also returned as a string.
Test Data:
("11", "10") -> "110"
("17", "19") -> "323"
("1", "0") -> "0"
("0", "0") -> "0"
Sample Solution:
JavaScript Code:
/**
* Function to multiply two integers represented as strings.
* @param {string} n1 - The first integer as a string.
* @param {string} n2 - The second integer as a string.
* @returns {string} - The product of the two integers as a string.
*/
function test(n1, n2) {
if (n1 === '0' || n2 === '0') // If either input is '0', return '0'
return '0';
n1_len = n1.length; // Length of first integer
n2_len = n2.length; // Length of second integer
result = new Array(n1_len + n2_len).fill(0); // Array to store the result
// Multiplication algorithm using arrays
for (i = n1_len - 1; i >= 0; i--) {
for (j = n2_len - 1; j >= 0; j--) {
t1 = i + j; // Index for tens place
t2 = i + j + 1; // Index for ones place
total = result[t2] + Number(n1[i]) * Number(n2[j]); // Calculate the product and add to the existing value
result[t2] = total % 10; // Store the ones place of the product
result[t1] += Math.floor(total / 10); // Store the carry-over to the tens place
}
}
if (result[0] === 0) // Remove leading zeros
result.shift();
return result.join(''); // Convert array to string and return the product
}
n1 = "11";
n2 = "10";
console.log("n1 = " + n1 + ", n2 = " + n2);
console.log("Product of the said two integers as strings: " + test(n1, n2));
n1 = "17";
n2 = "19";
console.log("n1 = " + n1 + ", n2 = " + n2);
console.log("Product of the said two integers as strings: " + test(n1, n2));
n1 = "1";
n2 = "0";
console.log("n1 = " + n1 + ", n2 = " + n2);
console.log("Product of the said two integers as strings: " + test(n1, n2));
n1 = "0";
n2 = "0";
console.log("n1 = " + n1 + ", n2 = " + n2);
console.log("Product of the said two integers as strings: " + test(n1, n2));
Output:
n1 = 11, n2 = 10 Product of the said two integers as strings: 110 n1 = 17, n2 = 19 Product of the said two integers as strings: 323 n1 = 1, n2 = 0 Product of the said two integers as strings: 0 n1 = 0, n2 = 0 Product of the said two integers as strings: 0
Flowchart:
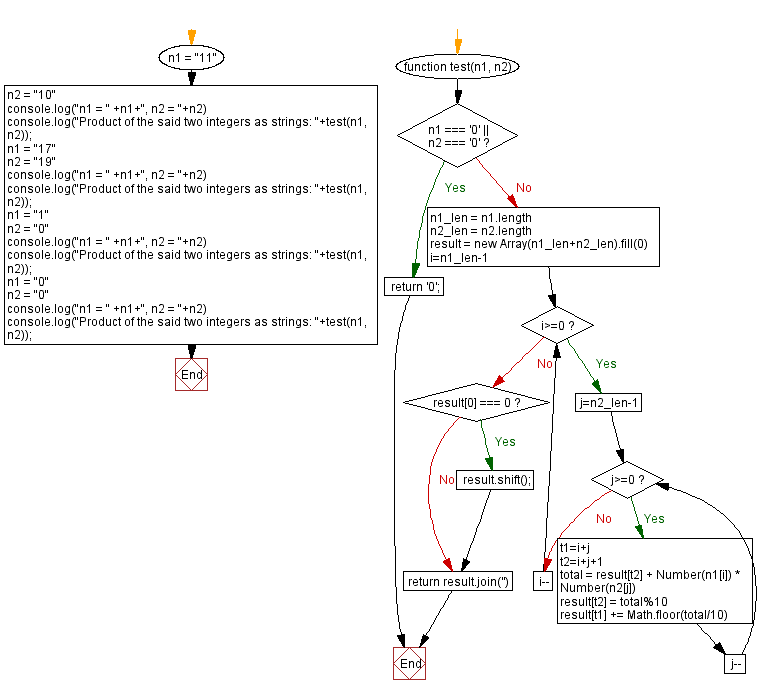
Live Demo:
See the Pen javascript-math-exercise-103 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that multiplies two non-negative integer strings by simulating the manual multiplication algorithm.
- Write a JavaScript function that handles carry-over during digit-by-digit multiplication and returns the product as a string.
- Write a JavaScript function that iterates over the digits of each string and computes the intermediate products before summing them.
- Write a JavaScript function that validates the input strings and returns "0" if either represents zero.
Improve this sample solution and post your code through Disqus.
Previous: Count total number of 1s from 1 to N.
Next: Distinct ways to climb the staircase.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.