JavaScript: Find the nth ugly number
JavaScript Math: Exercise-101 with Solution
Find nth Ugly Number
Write a JavaScript program to find the nth ugly number.
Ugly numbers are positive numbers whose only prime factors are 2, 3 or 5. The first 10 ugly numbers are:
1, 2, 3, 4, 5, 6, 8, 9, 10, 12, ...
Note: 1 is typically treated as an ugly number.
Visual Presentation:
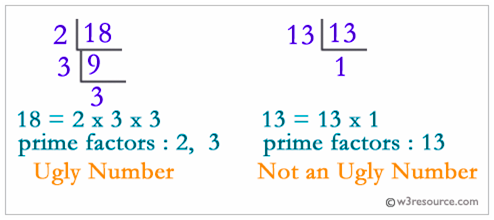
Test Data:
(4) -> 4
(10) -> 12
Sample Solution:
JavaScript Code:
/**
* Function to find the nth ugly number.
* @param {number} num - The number of ugly numbers to find.
* @returns {number} - The nth ugly number.
*/
function test(num) {
result = [1]; // Initialize the array with the first ugly number, which is 1
x2 = x3 = x5 = 0; // Initialize pointers for multiples of 2, 3, and 5
while (result.length < num) { // Continue until the array contains the specified number of ugly numbers
m2 = result[x2] * 2; // Calculate the next multiple of 2
m3 = result[x3] * 3; // Calculate the next multiple of 3
m5 = result[x5] * 5; // Calculate the next multiple of 5
temp = Math.min(m2, m3, m5); // Find the smallest among the calculated multiples
if (temp === m2) { // If the smallest is the multiple of 2, move to the next multiple of 2
x2++;
}
if (temp === m3) { // If the smallest is the multiple of 3, move to the next multiple of 3
x3++;
}
if (temp === m5) { // If the smallest is the multiple of 5, move to the next multiple of 5
x5++;
}
result.push(temp); // Add the smallest ugly number to the result array
}
return result[num - 1]; // Return the nth ugly number
};
// Test cases
n = 4;
console.log("n = " + n);
console.log("nth Ugly number is " + test(n));
n = 10;
console.log("n = " + n);
console.log("nth Ugly number is " + test(n));
Output:
n = 4 nth Ugly number is 4 n = 10 nth Ugly number is 12
Flowchart:
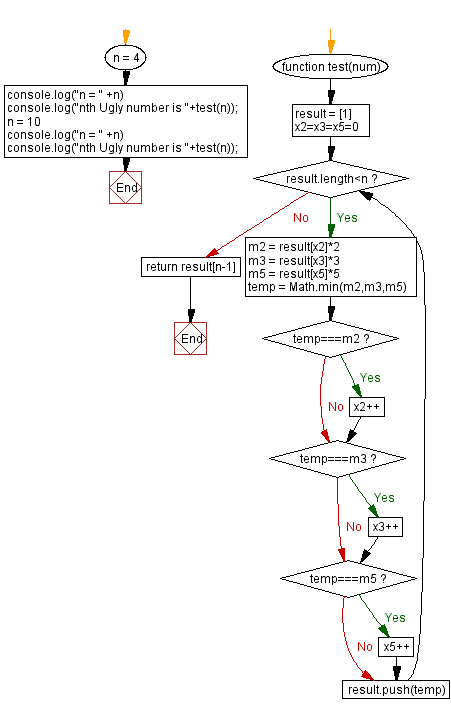
Live Demo:
See the Pen javascript-math-exercise-101 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that uses dynamic programming to generate ugly numbers and finds the nth ugly number.
- Write a JavaScript function that iteratively computes ugly numbers using multiple pointers and returns the nth element.
- Write a JavaScript function that stores computed ugly numbers in an array and retrieves the nth value efficiently.
- Write a JavaScript function that validates the input n and handles edge cases such as non-positive integers.
Improve this sample solution and post your code through Disqus.
Previous: Check a given number is an ugly number or not.
Next: Count total number of 1s from 1 to N.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.