JavaScript: Check a given number is an ugly number or not
JavaScript Math: Exercise-100 with Solution
Check Ugly Number
Write a JavaScript program to check if a given number is ugly.
Ugly numbers are positive numbers whose only prime factors are 2, 3 or 5. The first 10 ugly numbers are:
1, 2, 3, 4, 5, 6, 8, 9, 10, 12, ...
Note: 1 is typically treated as an ugly number.
Visual Presentation:
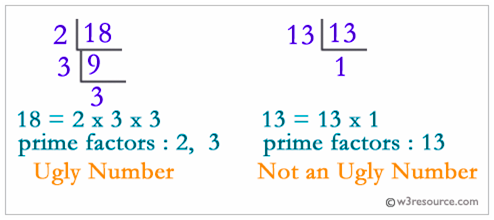
Test Data:
(12) -> true
(18) -> true
(19) -> false
Sample Solution:
JavaScript Code:
Output:
n = 12 Check the said number is an ugly number? true Original text: 18 Check the said number is an ugly number? true Original text: 19 Check the said number is an ugly number? false
Flowchart:
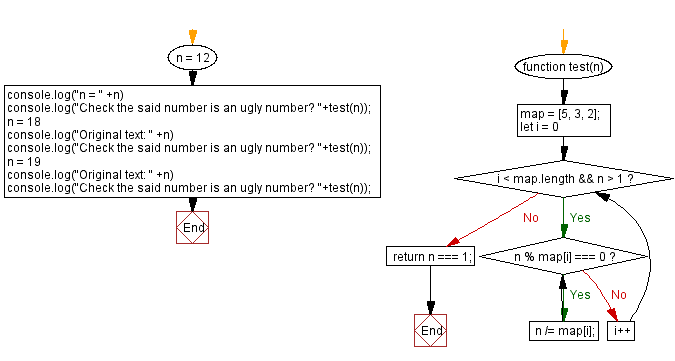
Live Demo:
For more Practice: Solve these Related Problems:
- Write a JavaScript function that checks if a number is ugly by repeatedly dividing it by 2, 3, and 5 until it equals 1.
- Write a JavaScript function that iterates through the prime factors 2, 3, and 5 to determine if a number is ugly.
- Write a JavaScript function that validates input and handles cases where the number is zero or negative when checking for ugliness.
- Write a JavaScript function that returns a boolean indicating if the number is ugly and logs intermediate division steps.
Go to:
PREV : Sum Digits Until One Digit.
NEXT : Find nth Ugly Number.
Improve this sample solution and post your code through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.