JavaScript: Convert a number from one base to another
JavaScript Math: Exercise-1 with Solution
Base Conversion Between Bases 2-36
Write a JavaScript function to convert a number from one base to another.
Sample Solution:
JavaScript Code:
var base_convert = function(number, initial_base, change_base) {
if (initial_base<2 || change_base<2 || initial_base>36 || change_base>36)
return 'Base between 2 and 36';
return parseInt(number + '', initial_base)
.toString(change_base);
}
console.log(base_convert('E164',16,8));
console.log(base_convert(1000,2,8));
console.log(base_convert(10,1,2));
console.log(base_convert(10,-2,2));
Output:
160544 10 Base between 2 and 36 Base between 2 and 36
Explanation:
In the exercise above,
- Function base_convert():
- The code defines a function named "base_convert()" which takes three parameters: 'number', 'initial_base', and 'change_base'. This function is designed to convert numbers from one base to another.
- Input validation:
- The function checks if either 'initial_base' or 'change_base' is less than 2 or greater than 36. If either condition is true, it returns the message 'Base between 2 and 36', indicating that the base should be within the valid range of 2 to 36.
- Number conversion:
- If the input bases are valid, the function converts the input 'number' to an integer using parseInt(number + '', initial_base). The "parseInt()" function parses a string argument and returns an integer of the specified radix (the 'initial_base' in this case).
- Then, it converts the obtained integer to a string representation in the desired base using .toString(change_base). This converts the number to the specified 'change_base'.
- The function returns the converted number as a string.
- Finally the code demonstrates two function calls using console.log():
- console.log(base_convert('E164', 16, 8));: This converts the hexadecimal number 'E164' to octal base (8) and prints the result.
- console.log(base_convert(1000, 2, 8));: This converts the decimal number 1000 from binary base (2) to octal base (8) and prints the result.
Flowchart:
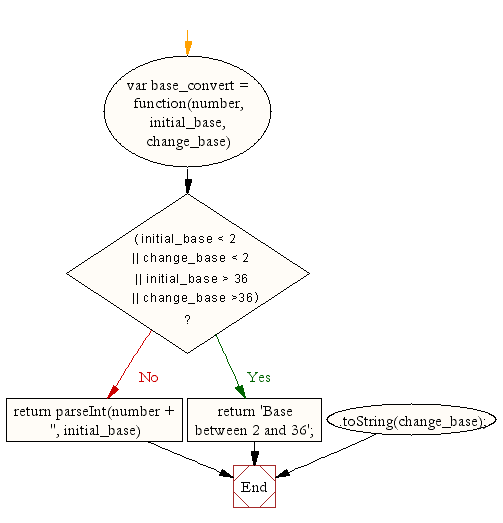
Live Demo:
See the Pen javascript-math-exercise-1 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus.
Previous: javascript Math Exercises
Next: Write a JavaScript function to convert a binary number to a decimal number.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics