JavaScript: Check a number is prime or not
JavaScript Function: Exercise-8 with Solution
Check Prime Using Recursion
Write a JavaScript function that accepts a number as a parameter and checks whether it is prime or not using recursion.
Note : A prime number (or a prime) is a natural number greater than 1 that has no positive divisors other than 1 and itself.
Visual Presentation:
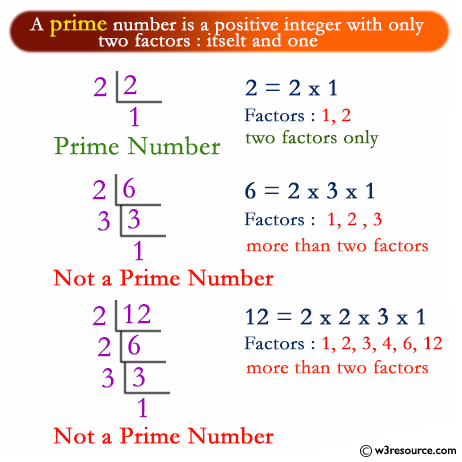
Sample Solution-1:
JavaScript Code:
// Define a function named test_prime that checks whether a given number n is a prime number
function test_prime(n) {
// Check if the number is equal to 1, which is not a prime number
if (n === 1) {
return false;
}
// Check if the number is equal to 2, which is a prime number
else if (n === 2) {
return true;
} else {
// Iterate from 2 to n-1 to check for factors of n
for (var x = 2; x < n; x++) {
// If n is divisible by x without a remainder, it is not a prime number
if (n % x === 0) {
return false;
}
}
// If no factors are found, the number is a prime number
return true;
}
}
// Log the result of calling test_prime with the input number 37 to the console
console.log(test_prime(37));
Output:
true
Flowchart:
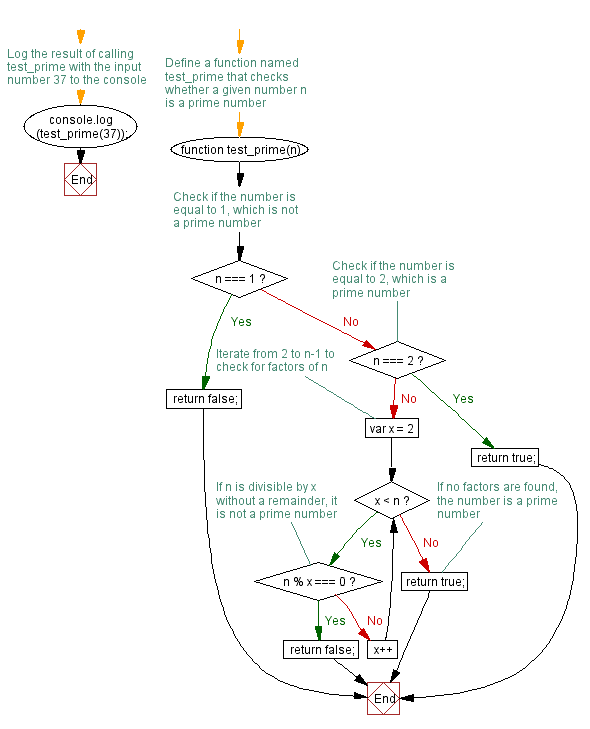
Live Demo:
See the Pen javascript-function-exercise-8 by w3resource (@w3resource) on CodePen.
Sample Solution-2:
JavaScript Code:
// Define a function named test_prime that checks whether a given number num is a prime number
function test_prime(num) {
// Check if the number is less than or equal to 1, as numbers less than or equal to 1 are not prime
if (num <= 1) {
return false;
}
// Iterate from 2 to the square root of num to check for factors
for (let i = 2; i <= Math.sqrt(num); i++) {
// If num is divisible by i without a remainder, it is not a prime number
if (num % i === 0) {
return false;
}
}
// If no factors are found, the number is a prime number
return true;
}
// Log the result of calling test_prime with the input number 37 to the console
console.log(test_prime(37));
// Log the result of calling test_prime with the input number 36 to the console
console.log(test_prime(36));
Output:
true false
Explanation:
The above function first checks if the input number is less than or equal to 1, which is not a prime number, and immediately returns false if it is. It then loops through all numbers from 2 to the square root of the input number using a for loop. It uses the modulus operator to check if each number is divisible by that number. If the input number is divisible by any number other than 1 and itself, it is not a prime number and the function returns false. Upon completion of the loop without finding a divisor, the function returns true, indicating that the input number is prime.
Flowchart:
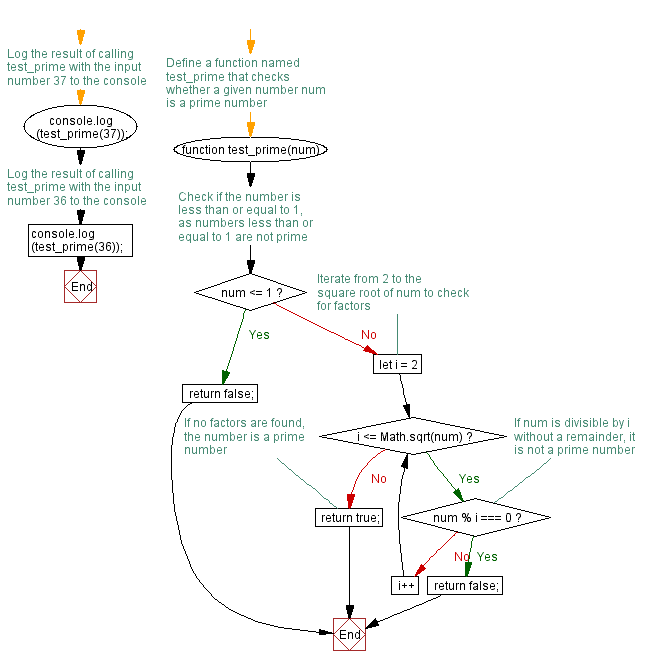
Live Demo:
See the Pen javascript-function-exercise-8-1 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that checks if a number is prime using recursion without employing any loops.
- Write a JavaScript function that recursively tests for factors and returns a boolean indicating whether the number is prime.
- Write a JavaScript function that implements a recursive prime check and correctly handles numbers less than 2.
- Write a JavaScript function that uses tail recursion to determine the primality of a given number.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript function that accepts a string as a parameter and counts the number of vowels within the string.
Next: Write a JavaScript function which accepts an argument and returns the type.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.