JavaScript: Counts the number of vowels within a string
JavaScript Function: Exercise-7 with Solution
Write a JavaScript function that accepts a string as a parameter and counts the number of vowels within the string.
Note : As the letter 'y' can be regarded as both a vowel and a consonant, we do not count 'y' as vowel here.
Sample Data and output:
Example string: 'The quick brown fox'
Expected Output: 5
Visual Presentation:
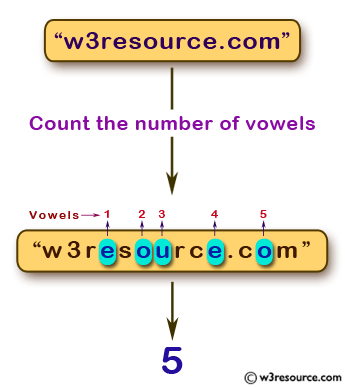
Sample Solution-1:
JavaScript Code:
// Define a function named vowel_count that takes a string parameter (str1)
function vowel_count(str1) {
// Define a string containing all the vowels in both lowercase and uppercase
var vowel_list = 'aeiouAEIOU';
// Initialize a variable vcount to count the number of vowels
var vcount = 0;
// Iterate through each character in the input string
for (var x = 0; x < str1.length; x++) {
// Check if the current character is a vowel by searching for it in the vowel_list
if (vowel_list.indexOf(str1[x]) !== -1) {
// If the character is a vowel, increment the vowel count
vcount += 1;
}
}
// Return the total count of vowels in the input string
return vcount;
}
// Log the result of calling vowel_count with the input string 'The quick brown fox' to the console
console.log(vowel_count("The quick brown fox"));
Output:
5
Explanation:
The indexOf() method returns the index within the calling String object of the first occurrence of the specified value, starting the search at fromIndex. Returns -1 if the value is not found.
Syntax -> str.indexOf(searchValue[, fromIndex])
Parameters :
searchValue : A string representing the value to search for.
fromIndex(Optional)-> The index at which to start the searching forwards in the string. It can be any integer.
The character(s) of the string will be counted as vowel if the condition (vowel_list.indexOf(str1[x]) !== -1) matched.
Flowchart:
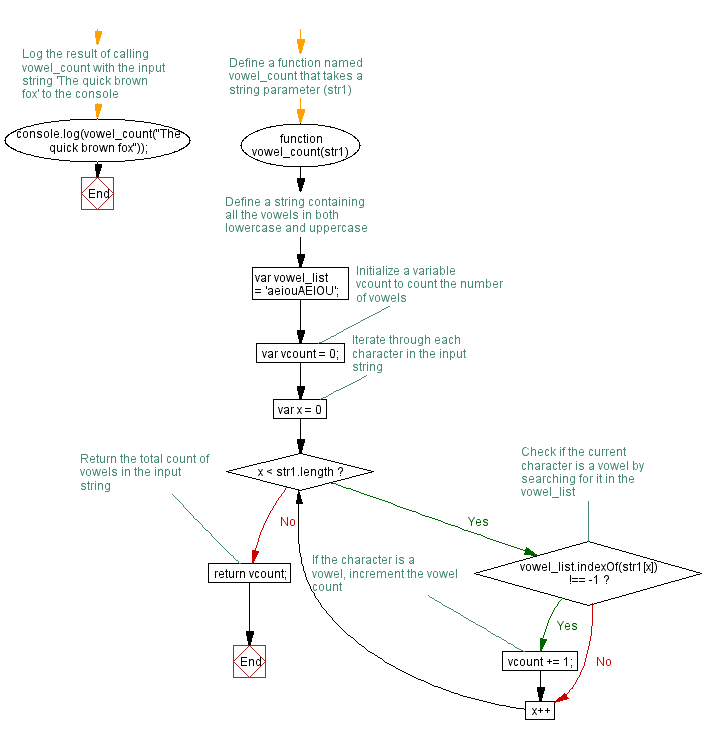
Live Demo:
See the Pen JavaScript -Counts the number of vowels within a string-function-ex- 7 by w3resource (@w3resource) on CodePen.
Sample Solution-2:
JavaScript Code:
function countVowels(str) {
// Use a regular expression to match all vowels in the string
const vowelMatches = str.match(/[aeiouAEIOU]/g);
// Return the count of vowels (null check in case no vowels are found)
return vowelMatches ? vowelMatches.length : 0;
}
// Example usage:
const inputString = 'The quick brown fox';
const result = countVowels(inputString);
console.log('Number of vowels in "${inputString}": ${result}');
Output:
Number of vowels in "The quick brown fox": 5
Flowchart:
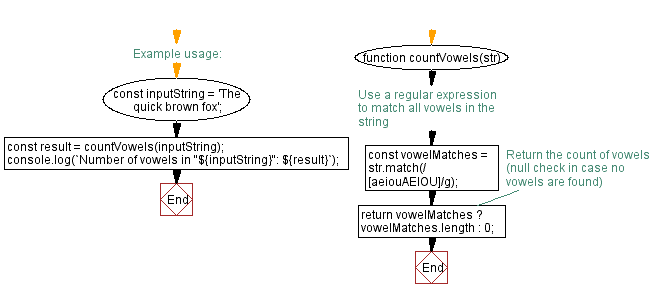
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript function that accepts a string as a parameter and find the longest word within the string.
Next: Write a JavaScript function that accepts a number as a parameter and check the number is prime or not.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/javascript-exercises/javascript-function-exercise-7.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics