JavaScript: Find the longest word within a string
JavaScript Function: Exercise-6 with Solution
Find Longest Word
Write a JavaScript function that accepts a string as a parameter and finds the longest word within the string.
Sample Data and output:
Example string: 'Web Development Tutorial'
Expected Output: 'Development'
Visual Presentation:
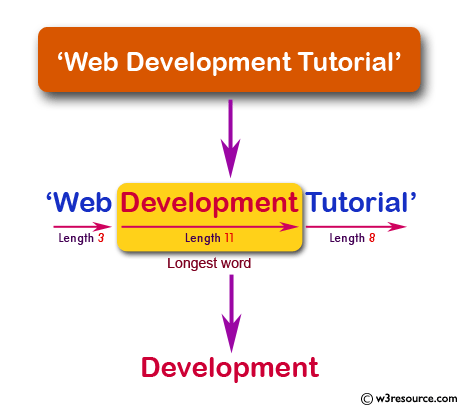
Sample Solution-1:
JavaScript Code:
// Define a function named find_longest_word that takes a string parameter (str)
function find_longest_word(str) {
// Use a regular expression to match words in the input string and store them in an array (array1)
var array1 = str.match(/\w[a-z]{0,}/gi);
// Initialize a variable result with the first word from the array
var result = array1[0];
// Iterate through the array of words starting from the second word
for (var x = 1; x < array1.length; x++) {
// Check if the length of the current word is greater than the length of the current result
if (result.length < array1[x].length) {
// If true, update the result with the current word
result = array1[x];
}
}
// Return the longest word found
return result;
}
// Log the result of calling find_longest_word with the input string 'Web Development Tutorial' to the console
console.log(find_longest_word('Web Development Tutorial'));
Output:
Development
Explanation:
Assume str = '@Web Development #Tutorial';
The match() method is used to retrieve the matches when matching a string against a regular expression.
Therefore str.match(/\w[a-z]{0,}/gi) will return ["Web", "Development", "Tutorial"].
For loop checks the length of the array element and compare with previous one and finally return the longest string.
The length property represents an unsigned, 32-bit integer that is always numerically greater than the highest index in the array.
Syntax -> arr.length
Flowchart:
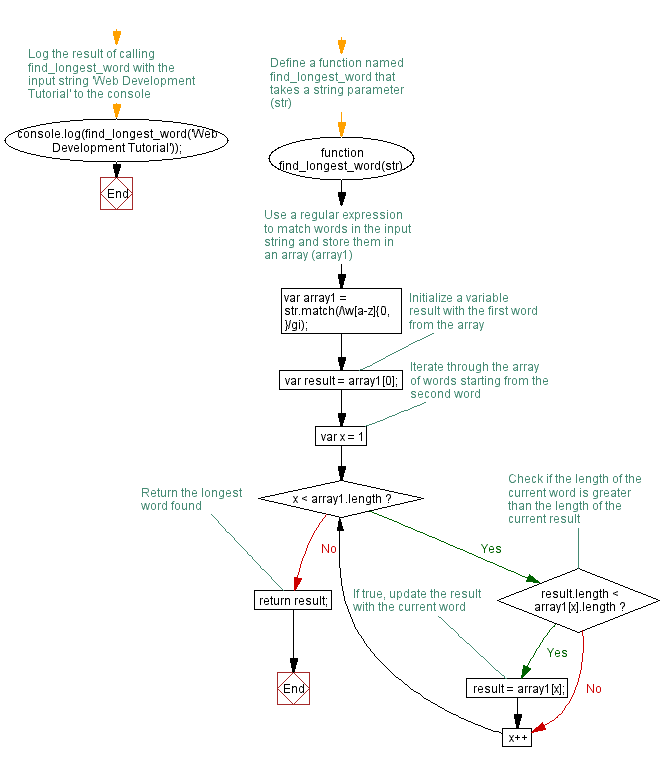
Live Demo:
See the Pen JavaScript -Converts the first letter of each word of a string in upper case-function-ex- 5 by w3resource (@w3resource) on CodePen.
Sample Solution-2:
JavaScript Code:
function find_longest_word(str) {
// Remove non-letter characters and convert to lowercase
const lettersOnly = str.replace(/[^a-zA-Z]/g, '').toLowerCase();
// Split the string into an array of words
const words = str.split(' ');
// Initialize a variable to store the longest word
let longest_Word = '';
// Loop through each word in the array
for (let i = 0; i < words.length; i++) {
// The longest word should be updated if the current word is longer than the longest word.
if (words[i].length > longest_Word.length) {
longest_Word = words[i];
}
}
// Return the longest word
return longest_Word;
}
const str = 'the quick brown fox';
console.log("The original string: "+str);
const result = find_longest_word(str);
console.log("The longest word of the said string: "+result)
console.log(result);
Output:
The original string: the quick brown fox The longest word of the said string: quick quick
Explanation:
The above find_longest_word() function takes a string as input and splits the string into an array of words using the split() method. It then loops through each word in the array and updates a variable result with the longest word found so far. Finally, the function returns the longest word.
The replace() method with a regular expression is used to remove any characters that are not letters before splitting the remaining letters into words. The regular expression /[^a-zA-Z]/g matches any character that is not a letter (i.e., any character that is not in the range a-z or A-Z), and the g flag makes the regular expression match all instances of non-letter characters in the string. In order to avoid affecting the comparison of word lengths, the resulting string is then converted to lowercase.
Flowchart:
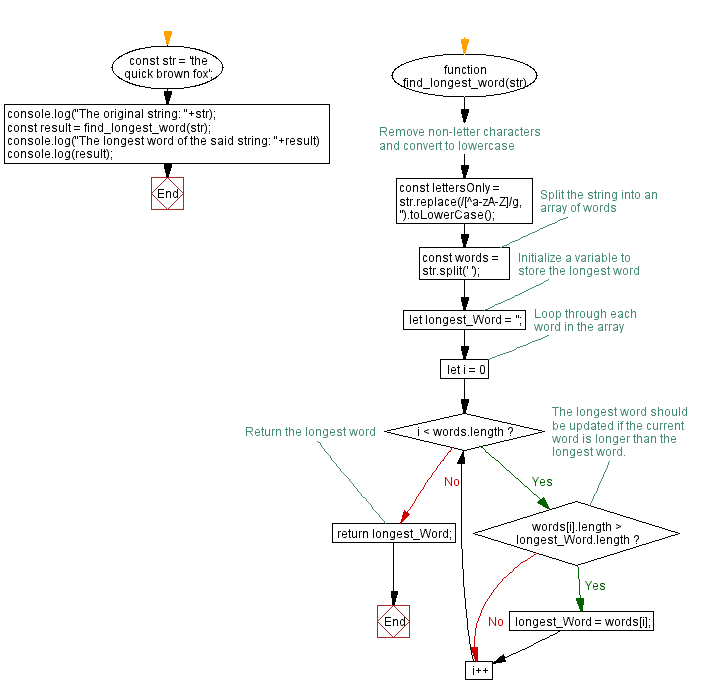
Live Demo:
See the Pen javascript-function-exercise-6-1 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that finds the longest word in a string, ignoring any punctuation.
- Write a JavaScript function that returns all words of maximum length from a given sentence.
- Write a JavaScript function that identifies the longest word in a string and returns its starting index.
- Write a JavaScript function that finds the longest word in a string using a recursive approach.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript function that accepts a string as a parameter and converts the first letter of each word of the string in upper case.
Next: Write a JavaScript function that accepts a string as a parameter and counts the number of vowels within the string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.