JavaScript: Converts the first letter of each word of a string in upper case
JavaScript Function: Exercise-5 with Solution
Capitalize First Letter of Each Word
Write a JavaScript function that accepts a string as a parameter and converts the first letter of each word into upper case.
Example string: 'the quick brown fox'
Expected Output: 'The Quick Brown Fox '
Visual Presentation:
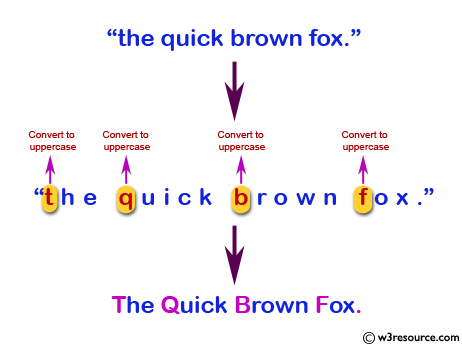
Sample Solution-1:
JavaScript Code:
// Write a JavaScript function that accepts a string as a parameter and converts the first letter of each word to uppercase.
function uppercase(str) {
// Split the input string into an array of words
var array1 = str.split(' ');
// Initialize an empty array to store the modified words
var newarray1 = [];
// Iterate through each word in the array
for (var x = 0; x < array1.length; x++) {
// Push the word with its first letter capitalized and the rest of the letters unchanged
newarray1.push(array1[x].charAt(0).toUpperCase() + array1[x].slice(1));
}
// Join the modified words into a single string, separated by spaces
return newarray1.join(' ');
}
// Log the result of calling uppercase with the input string "the quick brown fox" to the console
console.log(uppercase("the quick brown fox"));
Output:
The Quick Brown Fox
Explanation:
Assume str = "the quick brown fox";The split() method is used to split a String object into an array of strings by separating the string into substrings.
console.log(str.split(' '));
Output : ["the", "quick", "brown", "fox"]
First substrings -> "the"
Code to convert first character of the above sting to upper case-> array1[x].charAt(0).toUpperCase()
console.log(array1[x].charAt(0).toUpperCase()); [here x=0]
Output : "T"
Rest part of the string "the" -> array1[x].slice(1)
console.log(array1[0].slice(1));
Output : "he"
Final string :
console.log(array1[0].charAt(0).toUpperCase()+array1[0].slice(1));
Output : "The"
Now insert the above string into another array :
newarray1.push(array1[x].charAt(0).toUpperCase()+array1[x].slice(1));
Used functions :
The charAt() method is used to get the specified character from a string.
Syntax : str.charAt(index). Where index represents an integer between 0 and 1-less-than the length of the string.
The toUpperCase() method is used to convert the string value to uppercase.
The slice() method returns a shallow copy of a portion of an array into a new array object.
The push() method is used to add one or more elements to the end of an array and returns the new length of the array.
After completing the for loop return the final string :
return newarray1.join(' ');
The join() method joins all elements of an array into a string.
Flowchart:
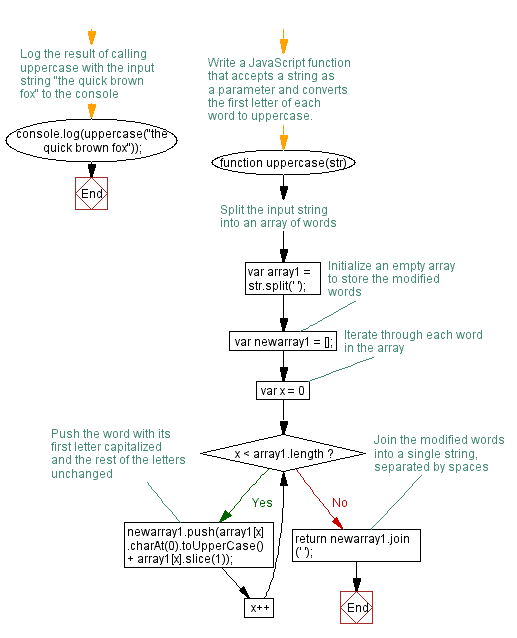
Live Demo:
See the Pen JavaScript -Returns a passed string with letters in alphabetical order-function-ex- 4 by w3resource (@w3resource) on CodePen.
Sample Solution-2:
JavaScript Code:
// Define a function named capitalize_First_Letter that takes a string parameter (text)
function capitalize_First_Letter(text) {
// Split the input string into an array of words
let words = text.split(" ");
// Iterate through each word in the array
for (let i = 0; i < words.length; i++) {
// Capitalize the first letter of each word and concatenate it with the rest of the word
words[i] = words[i].charAt(0).toUpperCase() + words[i].slice(1);
}
// Join the modified words into a single string, separated by spaces
return words.join(" ");
}
// Declare a constant variable text with the value 'the quick brown fox'
const text = 'the quick brown fox';
// Call the capitalize_First_Letter function with the input string 'the quick brown fox' and store the result in the variable result
const result = capitalize_First_Letter(text);
// Log the result to the console
console.log(result);
Output:
The Quick Brown Fox
Explanation:
This capitalize_First_Letter() function first splits the input string into an array of individual words using the split() method. To convert a word to uppercase, it uses the toUpperCase() method and charAt() to get the first letter.
The slice() method is then used to get the rest of the word after the first letter, which is then concatenated with the uppercase first letter to form the capitalized word. Finally, the capitalized words are joined back together into a single string using the join() method and returned
Flowchart:
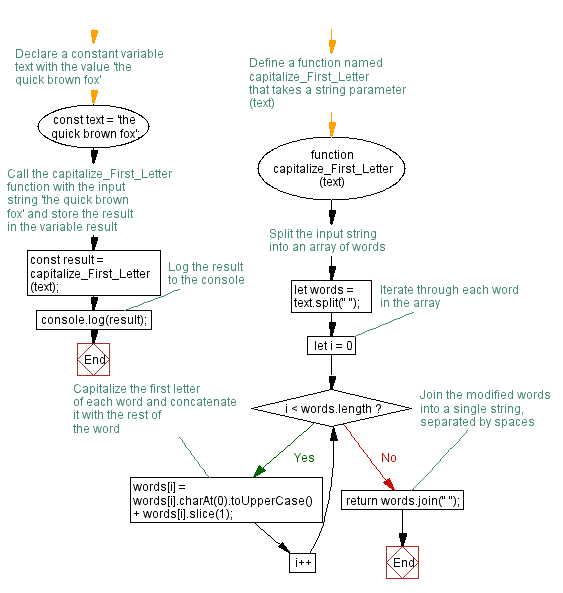
Live Demo:
See the Pen javascript-function-exercise-5-1 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that capitalizes the first letter of each word and converts the rest to lowercase.
- Write a JavaScript function that capitalizes the first letter of each word in a hyphen-separated string.
- Write a JavaScript function that capitalizes the first letter of each word in a string while handling multiple consecutive spaces gracefully.
- Write a JavaScript function that capitalizes the first letter of each sentence in a paragraph.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript function that returns a passed string with letters in alphabetical order.
Next: Write a JavaScript function that accepts a string as a parameter and find the longest word within the string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics