JavaScript: Returns a passed string with letters in alphabetical order
JavaScript Function: Exercise-4 with Solution
Sort String Alphabetically
Write a JavaScript function that returns a string that has letters in alphabetical order.
Example string: 'webmaster'
Expected Output: 'abeemrstw'
Note: Assume punctuation and numbers symbols are not included in the passed string..
Pictorial Presentation:
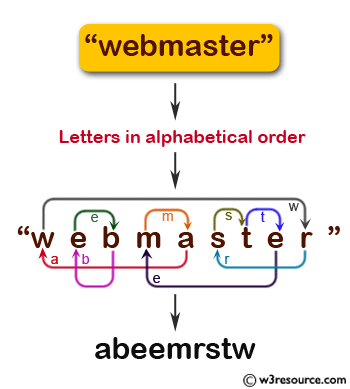
Sample Solution-1:
JavaScript Code:
// Define a function named alphabet_order that takes a string parameter (str)
function alphabet_order(str) {
// Split the string into an array of characters, sort the array, and join the characters back into a string
return str.split('').sort().join('');
}
// Log the result of calling alphabet_order with the input string "webmaster" to the console
console.log(alphabet_order("webmaster"));
Output:
abeemrstw
Explanation:
The split() method is used to split a String object into an array of strings by separating the string into substrings.Code : console.log('32243'.split(""));
Output : ["3", "2", "2", "4", "3"]
The sort() method is used to sort the elements of an array in place and returns the array.
Code : console.log(["3", "2", "2", "4", "3"].sort());
Output : ["2", "2", "3", "3", "4"]
The join() method is used to join all elements of an array into a string.
Code : console.log(["2", "2", "3", "3", "4"].join(""));
Output : "22334"
Flowchart:
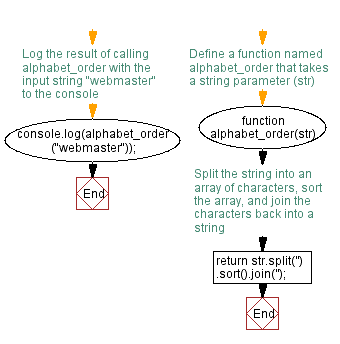
Live Demo:
See the Pen JavaScript -Returns a passed string with letters in alphabetical order-function-ex- 4 by w3resource (@w3resource) on CodePen.
Sample Solution-2:
JavaScript Code:
// Define a function named alphabet_order that takes a string parameter (str)
function alphabet_order(str) {
// Remove non-alphabetic characters from the input string using a regular expression
const lettersOnly = str.replace(/[^a-zA-Z]/g, '');
// Split the string of letters into an array, sort the array, and join the characters back into a string
const sorted = lettersOnly.split('').sort().join('');
// Return the sorted string
return sorted;
}
// Declare a constant variable str with the value 'web$mast@er'
const str = 'web$mast@er';
// Call the alphabet_order function with the input string 'web$mast@er' and store the result in the variable result
const result = alphabet_order(str);
// Log the result to the console
console.log(result);
Output:
abeemrstw
Explanation:
Using a regular expression, you can remove non-letter characters before sorting letters. The above function uses the replace() method with a regular expression to remove any characters that are not letters before sorting the remaining letters. The regular expression /[^a-zA-Z]/g matches any character that is not a letter (that is, any character that is not in the range a-z or A-Z), with the g flag making the regular expression match all instances of non-letter characters.
Flowchart:
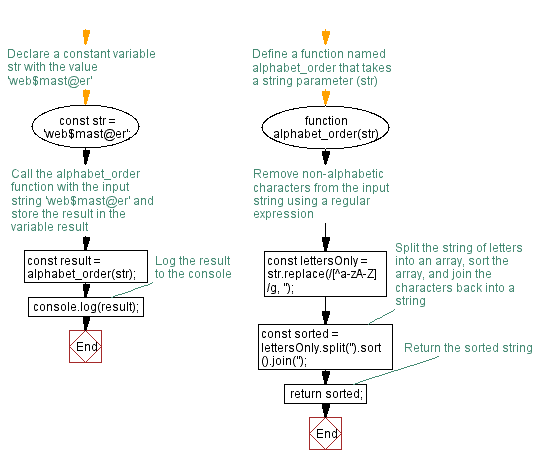
For more Practice: Solve these Related Problems:
- Write a JavaScript function that sorts the characters of a string in descending alphabetical order.
- Write a JavaScript function that sorts each word in a sentence alphabetically while preserving the original word order.
- Write a JavaScript function that sorts a string such that vowels appear before consonants in alphabetical order.
- Write a JavaScript function that alphabetically sorts a string's characters without using any built-in sort functions.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript function that generates all combinations of a string.
Next: Write a JavaScript function that accepts a string as a parameter and converts the first letter of each word of the string in upper case.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.