JavaScript: Pass a JavaScript function as parameter
JavaScript Function: Exercise-28 with Solution
Pass Function as Parameter
Write a JavaScript program to pass a 'JavaScript function' as a parameter.
Sample Solution-1:
JavaScript Code:
// Define a function named addStudent that takes an id and a refreshCallback function as parameters
function addStudent(id, refreshCallback) {
// Call the refreshCallback function
refreshCallback();
}
// Define a function named refreshStudentList that logs 'Hello' to the console
function refreshStudentList() {
console.log('Hello');
}
// Call the addStudent function with parameters 1 and the refreshStudentList function
addStudent(1, refreshStudentList);
Output:
Hello
Flowchart:
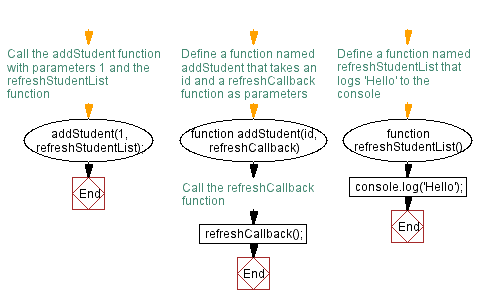
Live Demo:
See the Pen JavaScript - Pass a JavaScript function as parameter-function-ex- 28 by w3resource (@w3resource) on CodePen.
Sample Solution-2:
JavaScript Code:
// Function that takes a JavaScript function as a parameter
function executeFunction(func) {
console.log("Executing the provided function...");
func(); // Invoke the provided function
}
// Function to be passed as a parameter
function sampleFunction() {
console.log("This is a sample function.");
}
// Calling the main function and passing the sample function as a parameter
executeFunction(sampleFunction);
Output:
Executing the provided function... This is a sample function.
Flowchart:
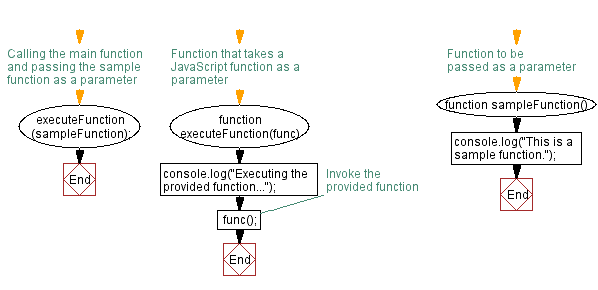
Improve this sample solution and post your code through Disqus.
Previous:Write a JavaScript function that returns the longest palindrome in a given string.
Next: Write a JavaScript function to get the function name.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics